Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial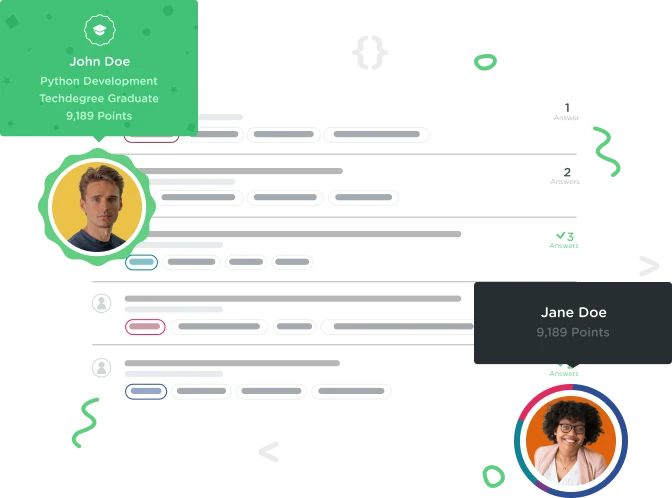

Abu Bakar Siddiq
4,673 Pointsi can not understand what is the logical problem in my code. can anyone help me?
i can not understand what is the logical problem in my code. can anyone help me? the question is ......
Write a method inside the Frog class named EatFly. It should take a single integer parameter named distanceToFly and return a bool value. It should return true if the frog can reach the fly with its tongue, and false otherwise.
namespace Treehouse.CodeChallenges
{
class Frog
{
public readonly int TongueLength;
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
public bool EatFly(int distanceToFly)
{
bool isReach = TongueLength > 0 && TongueLength == distanceToFly;
return isReach;
}
}
}
2 Answers
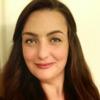
Jennifer Nordell
Treehouse TeacherHi there! While the code in the alternate answer will work, it's a bit verbose and the if/else statement is not actually required. it's generally considered better to return a boolean value directly. Ideally, you want to avoid a situation where you see "if this is true return true, otherwise return false". When this happens, you should be looking for a way to directly return the result of the evaluation of the expression.
This was my solution:
public bool EatFly(int distanceToFly)
{
return TongueLength >= distanceToFly;
}
This code asks the question if the the length of the tongue of the frog is greater than the distance to the fly. It will return the true or false value based upon that comparison.
Hope this helps!
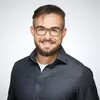
Raffael Dettling
32,998 PointsYou just need an if else statment in the EatFly method
--spoiler--
namespace Treehouse.CodeChallenges
{
class Frog
{
public readonly int TongueLength;
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
public bool EatFly(int distanceToFly)
{
if(distanceToFly <= TongueLength)
{
return true;
}
else
{
return false;
}
}
}
}
Raffael Dettling
32,998 PointsRaffael Dettling
32,998 PointsOh right forgot about this simple possibility :)