Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial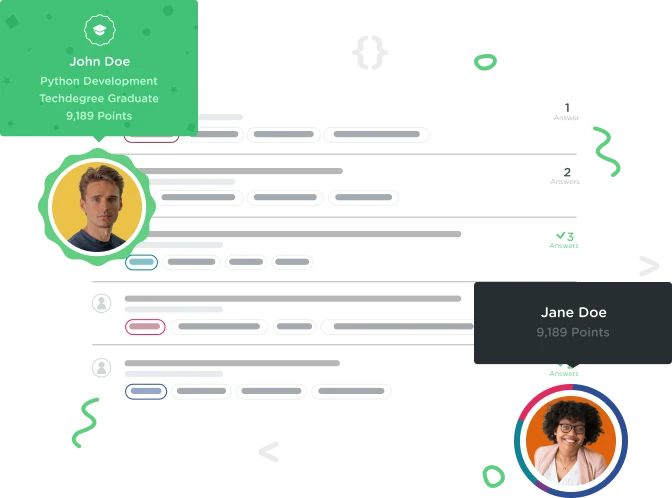
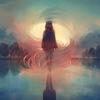
Aananya Vyas
20,157 Pointsi can not understand why 'selection' isn't recognised and the error isn't going away. please help.
//CODE SNIPPET:
// VendingMachine.swift
// VendingMachine
//
// Created by Aananya on 05/03/17.
// Copyright © 2017 Treehouse Island, Inc. All rights reserved.
//
import Foundation
enum VendingSelection {
case soda
case dietSoda
case chips
case cookie
case sandwich
case wrap
case candyBar
case popTart
case water
case fruitJuice
case sportsDrink
case gum
}
protocol VendingItem {
var price : Double {get}
var quantity: Int {get set}
}
protocol VendingMachine {
var selection : [VendingSelection] {get}
var inventory : [VendingSelection : VendingItem] {get set}
var amountDeposited : Double {get set}
init(inventory : [VendingSelection : VendingItem])
func vend(_ quantity : Int , _ selection : VendingSelection) throws
func deposit(_amount:Double)
}
struct Item {
let price : Double
var quantity : Int
}
enum InventoryError : Error {
case invalidResourse
case conversionFailure
case invalidSelection
}
class PlistConverter {
static func dictionary (fromFile name : String , ofType type : String) throws -> [String : AnyObject]{
guard let path = Bundle.main.path(forResource: name, ofType: type) else {
throw InventoryError.invalidResourse
}
guard let dictionary = NSDictionary(contentsOfFile : path) as? [String:AnyObject] else {
throw InventoryError.conversionFailure
}
return dictionary
}
class InventoryUnarchiver {
static func vendingInventory (fromDictionary dictionary : [String:AnyObject]) throws -> [VendingSelection:VendingItem] {
var inventory: [VendingSelection:VendingItem] = [:]
for(key , value) in dictionary {
if let itemDictionary = value as? [String:Any] ,
let price = itemDictionary ["price"] as? Double ,
let quantity = itemDictionary ["quantity"] as? Int {
let item = Item(price : price , quantity:quantity)
guard let selection = VendingSelection(rawValue : key) else {
throw InventoryError.invalidSelection}
}
inventory.updateValue(Item, forKey: selection)
}
}
}
} ```
4 Answers
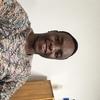
Kayondo Martin
7,481 Pointssame problem here, says VendingSelection can not be constructed because it has no accessible initializers
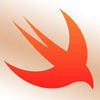
Jeff McDivitt
23,970 PointsCould you give me a little more information on the error?
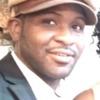
Chad Murdock
9,101 PointsAt a glance, it seems your return from the PlistConverter vendingInventory function doesn't exist and it needs to... try: return inventory just before the function's closing curly brace.

Chelsea Jackson
iOS Development with Swift Techdegree Student 130 Points"selection" isn't recognized because your enum cases do not yet have raw values. You need to set the type for the enum as "String", which will default every case's raw value to the string name, and it should work.