Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial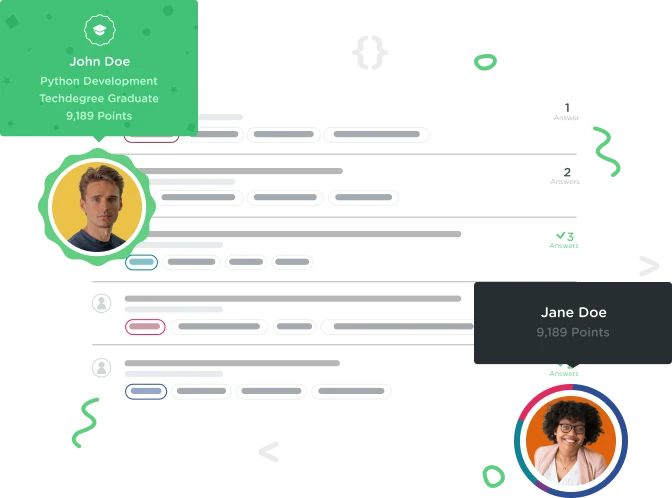
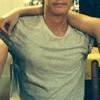
William Hartvedt Skogstad
5,857 PointsI canΒ΄t get the initializer method right..
In the editor, I have provided a class named Vehicle.
Your task is to create a subclass of Vehicle, named Car, that adds an additional stored property numberOfSeats of type Int with a default value of 4.
Once you've implemented the Car class, create an instance and assign it to a constant named someCar.
class Vehicle {
var numberOfDoors: Int
var numberOfWheels: Int
init(withDoors doors: Int, andWheels wheels: Int) {
self.numberOfDoors = doors
self.numberOfWheels = wheels
}
}
// Enter your code below
class Car: Vehicle {
var numberOfSeats: Int = 4
init(numberOfSeats: numberOfSeats) {
}
}
let someCare = Car(numberOfDoors: 4, numberOfWheels: 4, numberOfSeats: 4)
2 Answers
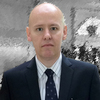
Nathan Tallack
22,159 PointsOk. There are two ways of going about this, and there are a few typo's you had that were probably making it difficult to work out.
The first (easy) way is this.
class Car: Vehicle {
var numberOfSeats: Int = 4
}
let someCar = Car(withDoors: 4, andWheels: 4)
We are not using an init method with your sublcass here because all of the properties you declare are either optional or have a default value (in your case the numberOfSeats has a default value). So what happens here when we declare your someCar we are using the initializer from the parent class (which is used if you dont define one in the subclass) to set the values up there in the parent class. Note that we are using the external parameter names when passing those values in. In this option we cannot declare your someCar with a different vlaue for numberOfSeats and can only initialize the object with that default value. We can change it later if we like.
The second (harder) way is this.
class Car: Vehicle {
var numberOfSeats: Int = 4
init(withDoors doors: Int, andWheels wheels: Int, numberOfSeats seats: Int) {
self.numberOfSeats = seats
super.init(withDoors: doors, andWheels: wheels)
}
}
let someCar = Car(withDoors: 4, andWheels: 4, numberOfSeats: 4)
Here we are declaring an initializer in our subclass. We are taking in a new parameter and in the initializer we are setting that with self.numberOfSeats. In this way we can (and must) pass in the numberOfSeats when we declare our someCar but in this case we are just passing in 4 so that it is the same as the default. Note also that we are calling the parent class initializer with the super.init function (which you do after you set all your own subclass properties) and we are passing into it the parameters that our initializer took when the object is being created. Again, we are taking care to use the external parameter names here as declared in the parent initializer (which are the same as what I am using in the subclass initializer) to make things easier.
I hope this helps. Watch those typo's, and keep up the great work! :)

Rich Braymiller
7,119 PointsDon't you LOVE how the teacher leaves out much of this stuff? SMH
William Hartvedt Skogstad
5,857 PointsWilliam Hartvedt Skogstad
5,857 PointsThanks a lot for clearing that up! :)