Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial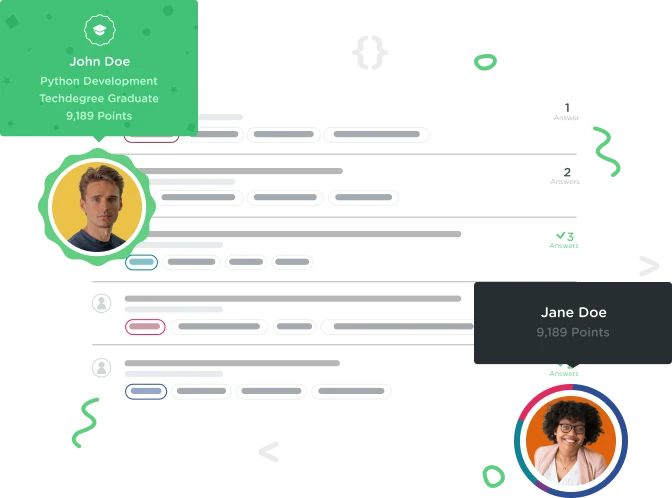

Alexandra Bonono
9,817 PointsI cannot complete the "Teamwork" challenge.
Hi,
Below are the main and prompter classes:
Main:
package com.teamtreehouse;
import java.io.IOException;
import java.util.List;
import java.io.*;
public class Main {
public static void main(String[] args) {
// write your code here
BufferedReader story = new BufferedReader(new InputStreamReader(System.in));
System.out.println("Please enter your story... ");
String newStory ="";
try {
newStory = story.readLine();
}catch (IOException e){
System.out.println("Couldn't load censored words");
e.printStackTrace();
}
System.out.printf("Your story is: %s%n", newStory);
//String story = "Thanks __name__ for helping me out. You are really a __adjective__ __noun__ and I owe you a __noun__.";
Template tmpl = new Template(newStory);
Prompter prompter = new Prompter();
try {
List<String> promptedTemplate = prompter.promptForWords(tmpl);
} catch (IOException e) {
e.printStackTrace();
}
prompter.run(tmpl);
}
}
Prompter:
package com.teamtreehouse;
import java.io.*;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.*;
public class Prompter {
private BufferedReader mReader;
private Set<String> mCensoredWords;
public Prompter() {
mReader = new BufferedReader(new InputStreamReader(System.in));
loadCensoredWords();
}
private void loadCensoredWords() {
mCensoredWords = new HashSet<String>();
Path file = Paths.get("resources", "censored_words.txt");
List<String> words = null;
try {
words = Files.readAllLines(file);
} catch (IOException e) {
System.out.println("Couldn't load censored words");
e.printStackTrace();
}
mCensoredWords.addAll(words);
}
public void run(Template tmpl) {
List<String> results = null;
try {
results = promptForWords(tmpl);
} catch (IOException e) {
System.out.println("There was a problem prompting for words");
e.printStackTrace();
System.exit(0);
}
String resultsOfSting = tmpl.render(results);
System.out.printf("Your TreeStory:%n%n%s", resultsOfSting);
}
/**
* Prompts user for each of the blanks
*
* @param tmpl The compiled template
* @return
* @throws IOException
*/
public List<String> promptForWords(Template tmpl) throws IOException {
List<String> words = new ArrayList<String>();
for (String phrase : tmpl.getPlaceHolders()) {
String word = promptForWord(phrase);
words.add(word);
}
return words;
}
/**
* Prompts the user for the answer to the fill in the blank. Value is guaranteed to be not in the censored words list.
*
* @param phrase The word that the user should be prompted. eg: adjective, proper noun, name
* @return What the user responded
*/
public String promptForWord(String phrase) throws IOException {
System.out.printf("Enter the '%s'.%n", phrase);
String response;
do{
System.out.println("Please type an uncensored word.");
response = mReader.readLine();
}while (mCensoredWords.contains(response));
System.out.printf("You typed %s%n", response);
return response;
}
}
Moderator edited: Added markdown so the code renders properly in the forums.
When I check my worl I get : " Bummer! Looks like you didn't present the TreeStory (3)" which is point 3. The user is presented with the completed story from the pseudo test. I thought I just need to print ou the story as a result? I am not sure what to do anymore. I am looking forward for your answers.
5 Answers
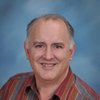
Mark Casavantes
Courses Plus Student 13,401 PointsGood Morning Alexandra,
On line one of Prompter change your import.java.util.; to import java.util.star; you are missing the star. The same is true on line one of Prompter with your import.java.io.;. Change it to import.java.io.star; You need to include the star or the specific part of the library like you did for the other libraries you imported.
When I posted my answer before the star would not show up. So I wrote the word "star", but I mean the symbol of shift and the 8 key. I am not sure why that is. Maybe someone could elaborate on this as well.
Bear with me. I am learning also but I think your code in public void run(Template tmpl) { needs to be in a new file. Maybe someone else can clarify this.
The method render may be missing in your program.
You may need one more } at the end of your Prompter program.
My answer is probably not complete. Make these corrections and repost. Also, other Treehouse students could add and modify my suggestions.
Thank you,
Mark
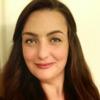
Jennifer Nordell
Treehouse TeacherHi Mark! As you can see, she has the java.util.*
. I'm curious as to why it's not showing up on your end. That being said, the reason you might not be able to get *
to show up on your posts is that these posts use markdown and the asterisk is a special character in markdown. If you have a spare hour one day, I can highly recommend taking this Markdown Basics course. Besides being able to make your answers better and more readable here on the forums, markdown is used in other places such as GitHub. It's a great way to make your GitHub repo really stand out with some snazzy markdown Thanks for helping out in the Community!

Alexandra Bonono
9,817 PointsThank you for your comment, I did not get error messages from imports I went back to have a look at my code but I still can't figure out whay os missing.

Alexandra Bonono
9,817 PointsHi Jennifer Nordell, thank you for your answer, I bookmarked the course, when I complete I will modify my comment. I too did not understand why my code was not showing up nicely like in other post.
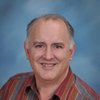
Mark Casavantes
Courses Plus Student 13,401 PointsGood Morning Alexandra,
Please post an update of your code and I will work on it.
Thank you,
Mark

Alexandra Bonono
9,817 PointsHi Mark Casavantes , I deleted the project by mistake, now I have downloded it again on my laptop and ran it before starting the coursework I did not modify anything and I get error messages from the prompter class. I have to say that I am stuck before even starting the coursework because I don't now what the error means. The first time I did the challenge I was working on another machine, I can't remmeber exatly what was different.
package com.teamtreehouse;
import java.io.*;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class Prompter {
private BufferedReader mReader;
private Set<String> mCensoredWords;
public Prompter() {
mReader = new BufferedReader(new InputStreamReader(System.in));
loadCensoredWords();
}
private void loadCensoredWords() {
mCensoredWords = new HashSet<String>();
Path file = Paths.get("resources", "censored_words.txt");
List<String> words = null;
try {
words = Files.readAllLines(file);
} catch (IOException e) {
System.out.println("Couldn't load censored words");
e.printStackTrace();
}
mCensoredWords.addAll(words);
}
public void run(Template tmpl) {
List<String> results = null;
try {
results = promptForWords(tmpl);
} catch (IOException e) {
System.out.println("There was a problem prompting for words");
e.printStackTrace();
System.exit(0);
}
// TODO:csd - Print out the results that were gathered here by rendering the template
}
/**
* Prompts user for each of the blanks
*
* @param tmpl The compiled template
* @return
* @throws IOException
*/
public List<String> promptForWords(Template tmpl) throws IOException {
List<String> words = new ArrayList<String>();
for (String phrase : tmpl.getPlaceHolders()) {
String word = promptForWord(phrase);
words.add(word);
}
return words;
}
/**
* Prompts the user for the answer to the fill in the blank. Value is guaranteed to be not in the censored words list.
*
* @param phrase The word that the user should be prompted. eg: adjective, proper noun, name
* @return What the user responded
*/
public String promptForWord(String phrase) {
// TODO:csd - Prompt the user for the response to the phrase, make sure the word is censored, loop until you get a good response.
return "";
}
}
Error message:
Information:java: Errors occurred while compiling module 'TreeStory2' Information:javac 9.0.1 was used to compile java sources Information:20/01/2018, 17:31 - Compilation completed with 1 error and 0 warnings in 4s 419ms /Users/admin/Downloads/local-development-environments/TreeStory/TreeStory2/src/com/teamtreehouse/Prompter.java Error:(27, 26) java: method readAllLines in class java.nio.file.Files cannot be applied to given types; required: java.nio.file.Path,java.nio.charset.Charset found: java.nio.file.Path reason: actual and formal argument lists differ in length
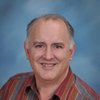
Mark Casavantes
Courses Plus Student 13,401 PointsGood Afternoon Alexandra,
I think the problems with your code comes from these two lines of code.
System.out.printf("Your TreeStory:%n%n%s", tmpl.render(results));
for (String phrase : tmpl.getPlaceHolders()) {
I could not identify your problem based on the error statement. I am sorry I have not yet been able to find a solution to your problem.
I hope this is helpful.
Mark

Daniel Phillips
26,940 PointsMaybe it's as easy as just printing the story without formatting.
Ace Belaro
19,607 PointsAce Belaro
19,607 PointsI am having the same problem :(