Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial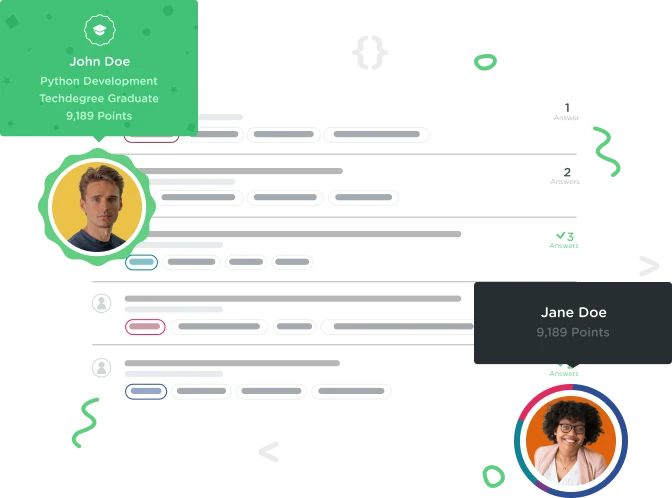

Austin Wyatt
3,146 PointsI cannot figure out how to add a method with a parameter of type double
I need to do the following: "We need to figure out the tax amount when the tax percentage is 7.5 percent. Call the method calculateTaxes on the variable item and assign the result to a new variable named taxes."
I have done the code that you see, but the coding challenge says that I need to add a method named calculateTaxes with a parameter named Percentage of type double. I'm not sure what I am doing wrong. If you could please help, that would be great!
struct Expense {
var description: String
var amount: Double = 0.0
init (description: String) {
self.description = description
}
func calculateTaxes(parameter: Double, percentage: Double) -> Double {
return (self.amount * (parameter/100))
}
}
var item = Expense(description: "Gas price")
item.amount = 100
var taxes = item.calculateTaxes(percentage)
// add the calculateTaxes method here
// it should accept only one parameter named 'percentage' of t
1 Answer
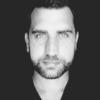
Jhoan Arango
14,575 PointsHello Austin :
What’s is important here is that you are very close and seem to understand most of the concept of accessing a structs properties and such with the dot syntax.
Please see the 2 codes below, the first one is yours, and I will highlight with comments some changes, and the second code will give you those changes and the answer to your challenge.
struct Expense {
var description: String
var amount: Double = 0.0
init (description: String) {
self.description = description
}
/*
The challenge says to create a method with a parameter named
“percentage” of type double. As you can see on your method,
you have an extra parameter.
(parameter: Double) should not be in the method’s signature.
*/
func calculateTaxes(parameter: Double, percentage: Double) -> Double {
return (self.amount * (parameter/100))
}
}
var item = Expense(description: "Gas price")
item.amount = 100
/*
Since you have an extra parameter not required by the challenge,
then you don’t need to add (percentage) here. Instead use a 7.5 which is
what the challenge wanted for taxes.
*/
var taxes = item.calculateTaxes(percentage)
This is what you want :
struct Expense {
var description: String
var amount: Double = 0.0
init (description: String) {
self.description = description
}
func calculateTaxes(percentage: Double) -> Double {
return (self.amount * (percentage / 100))
}
}
var item = Expense(description: "Gas Price" )
item.amount = 100
var taxes = item.calculateTaxes(7.5)
/*
You may be wondering, “Why did you not put item.calculateTaxes(percentage: 7.5)”
This is because the name of the parameter is not explicit. If you wanted to make
it explicit, you had to NAME your external parameter.
Examples:
on swift 1.2
func calculateTaxes(#percentage: Double) -> {}
on swift 2.0
func calculateTaxes(percentage percentage: Double) -> {}
*/
I hope that helps, if you have any other questions please ask :)
Happy learning