Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial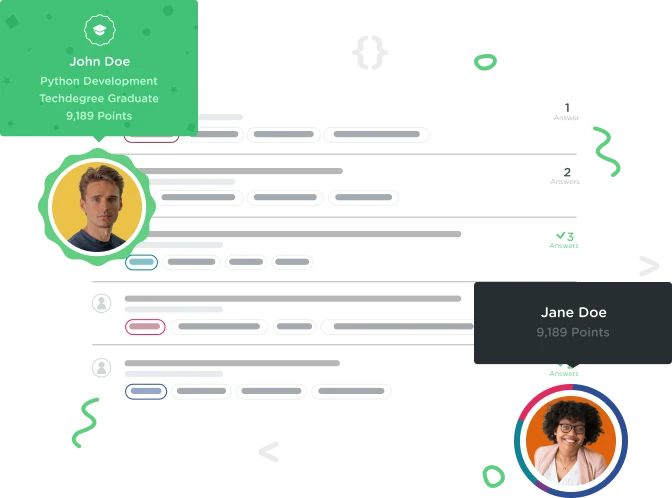
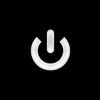
Joshua Reinhardt
17,732 PointsI CANNOT figure out how to get the function to see the argument as an array and evaluate the length.
I have tried approaching this code from every single direction. I am at the point where the function will return '0' if the argument is a number, string, or undefined. But when i follow up with the array.length command, it tells me that the argument (1, 2, 3) would print the result '0'. I don't get it. I don't get how to make the browser read the argument as an array with a definite length.
<!DOCTYPE html>
<html lang="en">
<head>
<title>JavaScript Foundations: Functions</title>
<style>
html {
background: #FAFAFA;
font-family: sans-serif;
}
</style>
</head>
<body>
<h1>JavaScript Foundations</h1>
<h2>Functions: Return Values</h2>
<script>
function arrayCounter(array) {
if(typeof array === "string", "number", "undefined"){
return 0}
return array.length;
}
</script>
</body>
</html>
6 Answers
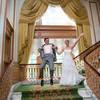
Colin Bell
29,679 PointsRemember these?:
|| === OR
&& === AND
So you want the statement to read if (type of array is "number" OR if type of array is "string" OR typeof is "undefined") then return zero. Else, return the length of array.
function arrayCounter(array) {
if (typeof array === 'number' || typeof array === 'string' || typeof array === 'undefined') {
return 0;
}
else {
return array.length;
}
}
I'm not sure if there is a shorthand for this or not.

Ryan Duchene
Courses Plus Student 46,022 PointsYou're really close. Try passing the object into the Array.isArray()
method instead:
if (Array.isArray(object)) {
// obj is an array
} else {
// obj is NOT an array
}
This is the way I know how to do it, at least. I hope that helps.
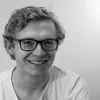
Luke Glazebrook
13,564 PointsHi Joshua!
A function like this can only return one value so the function actually gets stopped as soon as you return the 0 and continues with whatever else it has to do!
-Luke

Ryan Duchene
Courses Plus Student 46,022 PointsThe if
conditional will not let the return 0
statement run unless its condition is true. So the return array.length
statement should run if the condition is false.
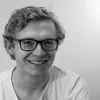
Luke Glazebrook
13,564 PointsOh, you're right! Sorry about that Joshua I obviously didn't examine your code well enough!
Take a look at Ryan Duchene's solution below!
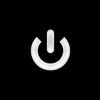
Joshua Reinhardt
17,732 PointsThanks for the help guys. This is my first venture into JS and it's a bit confusing. This has helped me wrap my head around things, but, sadly, the inclusion of the .isArray method gives me the message of the argument (1, 2, 3) coming out to zero. I want to say this is because 1, 2, 3 are all numbers, which will return 0 based on the first function command. But, this was by the instruction of the challenge, so I still don't know what to do.
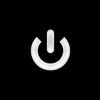
Joshua Reinhardt
17,732 PointsThanks for the help guys. This is my first venture into JS and it's a bit confusing. This has helped me wrap my head around things, but, sadly, the inclusion of the .isArray method gives me the message of the argument (1, 2, 3) coming out to zero. I want to say this is because 1, 2, 3 are all numbers, which will return 0 based on the first function command. But, this was by the instruction of the challenge, so I still don't know what to do.

Ryan Duchene
Courses Plus Student 46,022 PointsReverse the order of the conditional. Testing for an array will evaluate to true if the element is an array, and false if it is not.
if (Array.isArray(object)) {
// object must be an array
// return its length
return object.length;
} else {
// obj is NOT an array
// just return 0
return 0;
}
Here, rather than making sure that the object is NOT a number of string of something else, we're checking to see if it IS an array.
Hope that helps!
Joshua Reinhardt
17,732 PointsJoshua Reinhardt
17,732 PointsThat seemed to do the trick. Thank you!