Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial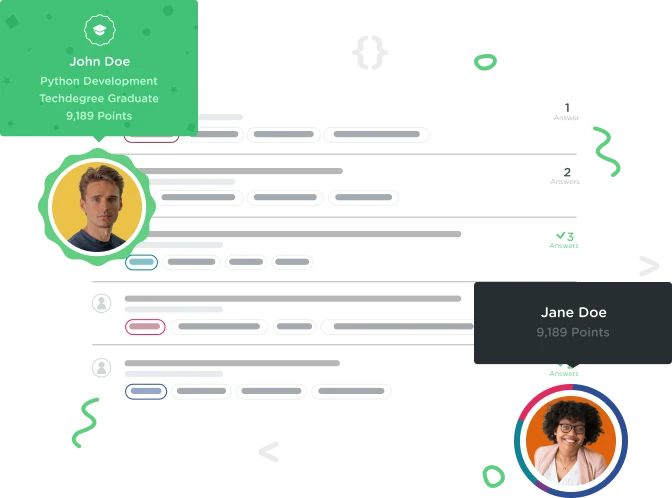

Cheri Watts
658 PointsI cannot figure out this quiz question. Why can't I get back to the video???
In the Challenge "Create a variable named full_name..." I cannot figure it out. Why can't I get back to the video training to watch it again?
full_name = "Cheri Watts"
name_list = list(full_name[])
5 Answers
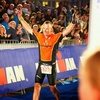
Steve Hunter
57,712 PointsHi there,
Your progress through each stage of a course is tracked in the (for this course) pink bar running across the top of the screen. Each tick is a video or challenge completed. If you hover over the tick, you can see which video lies behind it and you can then click through to it.
Steve.

Cheri Watts
658 PointsThx Steve.
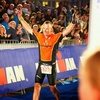
Steve Hunter
57,712 PointsNo problem! :-)
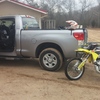
Ryan Ruscett
23,309 PointsHey,
You need a variable named full_name and you need to get that into a list and save it as name_list.
full_name = "Hello world"
I need to get this into a list. Now, you are taking a list contructor approach. By calling list on a string. Here watch what happens when you do this.
>>> aStr = "Hello my name is Ryan"
>>> lst = list(aStr)
>>> lst
['H', 'e', 'l', 'l', 'o', ' ', 'm', 'y', ' ', 'n', 'a', 'm', 'e', ' ', 'i', 's', ' ', 'R', 'y', 'a', 'n']
>>>
This is surely not what we are looking for. We want to split up a list based on the spaces. Not on each individual character. So how do we do that? Back to python
We can use dir() and help() to figure it out.
We have a string that needs to be split. So let's look at the string object.
>>> dir(string)
['__add__', '__class__', '__contains__', '__delattr__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__getitem__', '__getnewargs__', '__gt__', '__hash__', '__init__', '__iter__', '__le__', '__len__', '__lt__', '__mod__', '__mul__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__rmod__', '__rmul__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__', 'capitalize', 'casefold', 'center', 'count', 'encode', 'endswith', 'expandtabs', 'find', 'format', 'format_map', 'index', 'isalnum', 'isalpha', 'isdecimal', 'isdigit', 'isidentifier', 'islower', 'isnumeric', 'isprintable', 'isspace', 'istitle', 'isupper', 'join', 'ljust', 'lower', 'lstrip', 'maketrans', 'partition', 'replace', 'rfind', 'rindex', 'rjust', 'rpartition', 'rsplit', 'rstrip', 'split', 'splitlines', 'startswith', 'strip', 'swapcase', 'title', 'translate', 'upper', 'zfill']
>>>
Hmm I see split. But I don't know what split does? I can use help now on string.split().
>>> help(string.split)
Help on built-in function split:
split(...) method of builtins.str instance
S.split(sep=None, maxsplit=-1) -> list of strings
Return a list of the words in S, using sep as the
delimiter string. If maxsplit is given, at most maxsplit
splits are done. If sep is not specified or is None, any
whitespace string is a separator and empty strings are
removed from the result.
>>>
Hey that looks like exactly what I need. It takes a sep or seperation argument and returns a list of strings. Ok so I know from the directions I want to split on the spaces. So let's try that
>>> lst2 = aStr.split(" ")
>>>lst2
['Hello', 'my', 'name', 'is', 'Ryan']
Ok awesome. This is exactly what I need. A list of the individual words in a string. Since split returns a string. I don't need to convert it to a string. Just save it as a variable. Like so
full_name = "Hello World"
name_list = full_name.split(" ")
I use the quotes with a space in between for the separation. Saying i want to split the string up on spaces. If I don't include the open space. Than I get the same result when I call the list(aStr) which means it by default splits on every character in a sequence including white spaces.
I hope this helps. Please let me know if something is unclear and I will help you through it!

Cheri Watts
658 PointsRyan,
Thank you so much!
Here is what I have for the Challenge that I am engaging:
full_name = "Cheri Watts" name_list = full_name.split() greeting_list = ["Hi", "I'm Treehouse"()]
When I do this, I get Oops looks like Challenge 1 is no longer passing...
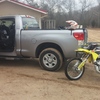
Ryan Ruscett
23,309 PointsHey,
Sure no problem. Let's see if we can clear this up for you. Ok. So let's walk through this.
full_name = "Cheri Watts"
name_list = full_name.split()
greeting_list = ["Hi", "I'm Treehouse"()] <---------- HERE
greeting_list is suppose to be a a string. So we define a string like this.
greeting_list = "Hi, I'm Treehouse"
That is the first thing to do. Now we need to look at the second part. I need to take that string and make it into a list. That is split on the spaces. Just like we did previously. There are two ways to accomplish this.
greeting_list_to_convert = "Hi, I'm Treehouse"
greeting_list = greeting_list_to_convert.split()
OR - we can use the literal string and split that. I'll explain below.
greeting_list = "Hi, I'm Treehouse".split()
You are trying to put a string into a list and use (). Split returns a list. So no need to put the string into the list. You also don't need (). () is used to when calling a function or method. There are times where you leave those off when calling a function or method. But we wont get into that here.
You also used "" and ' quotes. Which is correct. I can put single or double quotes around a string. If the string has a single quote in it. Then I must surround my string with double quotes. If there are double quotes in my string than I will surround it with single quotes. Or if there are no double or single quotes in my string. I will use either single or double. Example below.
"Hi I'm Treehouse" <<<Have a single quote in the string so I surround it with double quotes.
'Hi "I love" pizza' ... double quotes in the string surround with single quotes.
'Hi there' <<< ok
"Hi there" <<< ok
A string reference is this.
a = "hi"
a - references the data of type string "hi" So I can pass the letter "a" around and it will be representing "hi"
Take print for example:
print(a)
"hi"
I could also print the literal string.
print("hi)
"hi"
I don't always recommend calling a function based off the literal. But in this case we can do it. I showed you two ways to accomplish the same task. Even a literal is still a string.
Does this help answer your question? Please let me know if I can help! Thanks!