Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial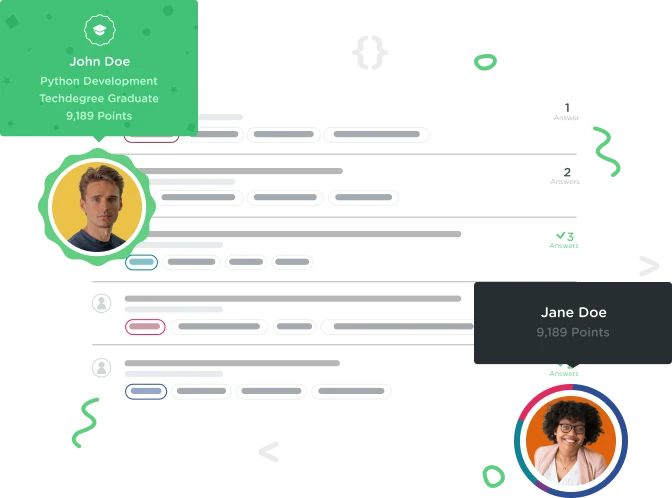

Sıla Çakıcı
371 PointsI cannot see what the solution to my problem on numberOfSides class is
I cannot see what the solution to my problem on numberOfSides class is. Could you help me?
// Enter your code below
let someShape
class Shape {
var numberOfSides: Int
init() {
}
}
2 Answers

Anjali Pasupathy
28,883 PointsYou're nearly there. You just need to fill out your init method and instantiate a shape.
In your init method, you need to assign a value to all properties that don't currently have a value. In this case, the only property of Shape that hasn't been assigned a value is numberOfSides. To assign numberOfSides a value, you need to pass in the number of sides as a parameter of the init method, and assign that parameter to the numberOfSides property in the body of the init method.
init(numberOfSides: Int) {
self.numberOfSides = numberOfSides
}
It's important to note that in this case, the call to self is necessary because if you just said "numberOfSides = numberOfSides", the compiler would think that you're setting the parameter numberOfSides equal to itself, rather than setting the property numberOfSides to the parameter numberOfSides. If you call the parameter something different, or use an internal parameter with a different name, the call to self isn't necessary:
// CALLING THE PARAMETER SOMETHING DIFFERENT
init(howManySides: Int) {
numberOfSides = howManySides
}
// USING AN INTERNAL PARAMETER WITH A DIFFERENT NAME
// The external parameter (numberOfSides) is the parameter we use when we're actually initializing the class Shape
// The internal parameter (sides) is the parameter we use in the body of the init method
init(numberOfSides sides: Int) {
numberOfSides = sides
}
After filling out your init method, all you have to do is instantiate a Shape object and store it in a constant called someShape:
let someShape = Shape(numberOfSides: 3) // or whatever number you want to use
Make sure the parameter you're using when you instantiate Shape is the same parameter you use in your init method.
I hope this helps!

Sıla Çakıcı
371 PointsOK, this solved my problem. Thank you for your helps!

Anjali Pasupathy
28,883 PointsYou're welcome! (: