Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial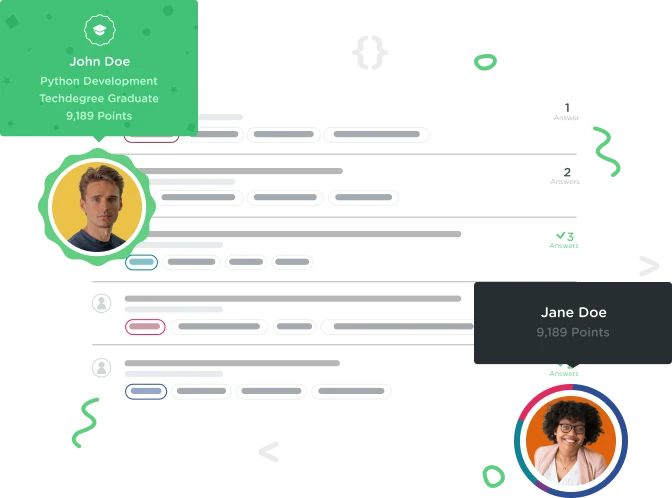

LauraAnn Drury
462 PointsI cannot seem to figure out part 2 of this challenge...
I'm trying to retrieve the sum for the numbers inside of the array and cannot seem to find how. This is the challenge associated with the while and repeat loops.
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
// Enter your code below
while counter < numbers.count {
print(numbers)
counter++
}
repeat {
print (numbers[index])
numbers++
} while counter < numbers.count
2 Answers

David Garner
4,181 PointsYou are close first you need to delete your repeat-while loop you only need one while loop for this task
Your code should look something like this:
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
// Enter your code below
while counter < numbers.count {
sum = sum + numbers[counter]
counter++
}
Above you access the item in the numbers array with the counter variable.
So when counter = 0 the value of numbers[counter] = 2
counter = 0 numbers[counter] = 2
counter = 1 numbers[counter] = 8
counter = 2 numbers[counter] = 1
counter = 3 numbers[counter] = 16
etc it will stop once counter is equal to the size of the numbers array
sum = sum + numbers[counter]
adds the previous sum together with the value of numbers[counter]
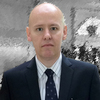
Nathan Tallack
22,159 PointsYour while counter was good for the first task in the challenge.
But for the second task they were asking you to compute the sum within the while loop. If you did that your code would end up looking something like this.
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
// Enter your code below
while counter < numbers.count {
print(numbers)
let newValue = numbers[counter] // Here we read the current number.
sum += newValue // Here we add the current number to the sum variable.
counter++
}