Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial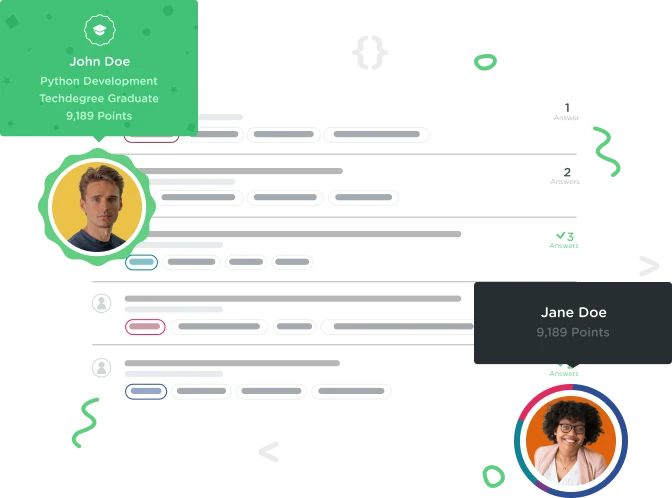

Christopher Borchardt
2,908 PointsI can't complete the squared challenge
This challenge is similar to an earlier one. Remember, though, I want you to practice! You'll probably want to use try and except on this one. You might have to not use the else block, though. Write a function named squared that takes a single argument. If the argument can be converted into an integer, convert it and return the square of the number (num ** 2 or num * num). If the argument cannot be turned into an integer (maybe it's a string of non-numbers?), return the argument multiplied by its length. Look in the file for examples.
That is the challenge. I've run the code in workspace and it works with all the test values listed. I've tried with just except ValueError, I've tried with just TypeError, both come back with errors that basically just say 'try again' If i use both of them, or just use "except:" in the code it comes back that 'squared' did not return the correct results.
# EXAMPLES
# squared(5) would return 25
# squared("2") would return 4
# squared("tim") would return "timtimtim"
def squared(num):
try:
int(num)
return num * num
except ValueError:
return num * len(num)
except TypeError:
return num * len(num)
3 Answers
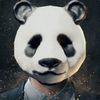
Haider Ali
Python Development Techdegree Graduate 24,728 PointsHi Christopher. In this challenge, it does not ask us to catch a specific error. Because of this, you should just write except
and it will catch any error that comes up:
def squared(num):
try:
num = int(num) #try to turn num into integer
return num * num #try to return num squared
except: #if an error comes up
return num * len(num) #just return num multiplied by its length
Thanks,
Haider
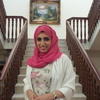
Assma Al-Adawi
8,723 PointsWhy can't I just use if and else in this challenge? I feel like it makes more sense and is more intuitive...

Kevin Smith
766 PointsHello, I hope you see this comment. In the comments you wrote "try to turn num into integer". If the user inputs a number shouldn't python recognize it as an integer? (I'm not implying you're wrong, I just don't get why you have to declare a number as an integer)
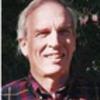
jcorum
71,830 PointsHere you go:
def squared(num):
try:
return (int(num) ** 2)
except ValueError:
return (str(num) * len(num))
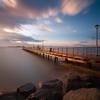
Qasa Lee
18,916 PointsCool as mine LOL!

Christopher Borchardt
2,908 PointsI have tried except, and it came back saying that the number was wrong (that the code returned a wrong value). For some reason it was not accepting num * num as a valid code. when i changed it back to num ** 2 it went through fine.
Daniel Escobar
2,580 PointsDaniel Escobar
2,580 Pointsit says to catch an exception but does not state if ValueError or TypeError. If you do it with just except, it would work just fine!