Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial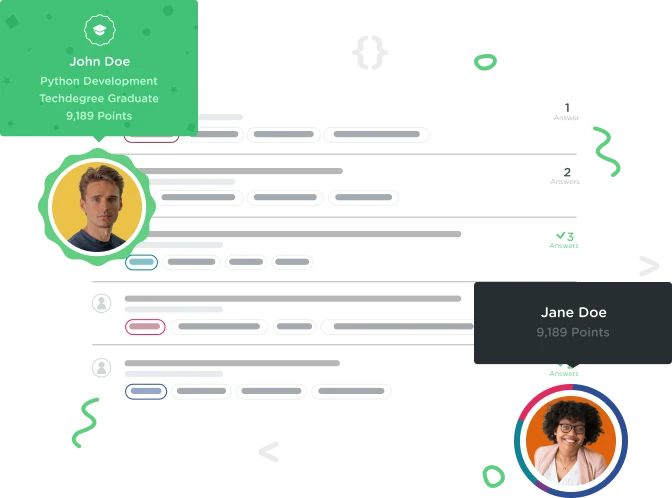
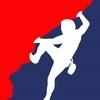
Greg King
4,073 PointsI can't discern the difference between method and object
I don't mean this literally in the sense that I can't conceptualize the two but, I mean that I don't know how to write or call an object versus a method. What tells the computer which is happening and what are the key differences that I'm supposed to be looking for?
2 Answers

Ken Alger
Treehouse TeacherGreg;
Welcome to Treehouse! Great question, let me see if I can shed some light on things without causing too much confusion.
To attempt to over simplify things for explanation purposes, there are three general things you need to grasp in object oriented programming.
1) Classes,
2) Methods, or sometimes called functions, and
3) Objects
A class is like a blue print for an object. Let's say that we make a class called dog
. There are lots of dogs, and although there are differences, they all follow the same design pattern. However, if you have a specific dog, that is an object. This one dog can be petted, it is real, it is not the blue prints but something that you can work with.
Methods allow you to define what the object does or can be done to it. So, you could have a pet()
method for your dog
object, a bark()
method, a tailWag()
method, etc. In our example when we create a dog
object in our code we would want to assign it a name at creation, make sense? That way we can make each dog
have different attributes and keep track of them.
In code we would do something like:
public class Dog { // Define our class
// Define our variables
private String mDogName;
public Dog(String dogName) { // define a method
mDogName = dogName; // sets our dog's name to the name passed in when we create a new dog object
}
}
Great! We can now create as many dog
objects we want and name them in our code with Dog("Fido")
or Dog("Rover")
. We would generally assign meaningful names to our dog objects as well along the lines of:
Dog fido = new Dog("Fido");
Dog rover = new Dog("Rover");
For what sounds like your main question then, looking at the previous code, Java will create new dog
objects Fido and Rover and assign their respective names based on the method we declared inside our class Dog
. That is what objects are, something you can use or do something to, they are "real".
Methods, as I said earlier, are something you do to your objects. Let's say that in our Dog
class we create another method like so:
public boolean pet() {
mDogIsHappy = true;
return mDogIsHappy;
}
Assuming that our variables are assigned, etc. we could now call a method on our dog object and have our dog be happy, right? We would do that with:
fido.pet();
We can obviously do much more (use more methods) than just pet our dog and we can assign it more properties (change our object) than name, but hopefully that helps a bit.
Please post back if you are still confused.
Ken

Andre Du Plessis
4,874 PointsAn object you would have to instantiate first (unless it is defined as static), before you can call it's methods.
Methods are basically actions you can call that is owned by the object. So from your Main code or other object code to for example hear a cat speak :
Cat cat = new Cat(); <--- instantiate object Cat
cat.speak(); <--- call method (also called a function as it returns a string "Meow")
Note : you only have access to the object's public methods in this manner.
If you are writing code inside the current object's code you can just call the method without prefixing the class name. For example :
public class Cat {
private int mMeals = 0;
public String speak(){
return "Meow";
}
private void fedToday(){
mMeals++;
}
public String FeedMyKitty(){
fedToday();
return speak();
}
}
So inside the object you can call both private and public methods.
Hope this helps.
Greg King
4,073 PointsGreg King
4,073 PointsThank you..this helps mucho
Jonathan Sanders
752 PointsJonathan Sanders
752 PointsI appreciate that this was answered two years ago, so this may not be picked up, but here goes!
Really useful explanation there. Looking at the first block of code in the answer above, the Dog method has in its parameter (String dogName). This makes sense to me, because it tells me that the method will accept a string when it's called, which it will assign to the instantiated object of the class Dog with a string variable mDogName. (correct so far?)
My question is, in the third code block in the answer given above, boolean is given as a return type for the method pet. Why isn't boolean mDogIsHappy contained in the parameters of pet? What's the difference between setting a return type to a method and setting its parameters?