Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial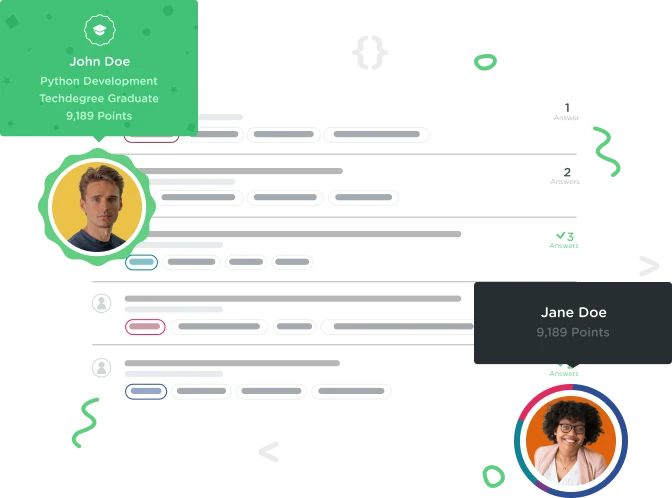
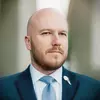
Jared Armes
Courses Plus Student 6,391 PointsI can't figure out how to find an index
I think the problem lies with the methodology of my "if" statement. I can't seem to figure out the syntax necessary to search the array for indexes. I know that I have been asking a lot of questions throughout this section, but it is starting to make sense, thanks to your help! Any input whatsoever would be greatly appreciated.
class TodoList
attr_reader :name, :todo_items
def initialize(name)
@name = name
@todo_items = []
end
def add_item(name)
todo_items.push(TodoItem.new(name))
end
def find_index(name)
index = 0
found = false
if todo_items(name)
found = true
return index
else
return nil
end
end
end
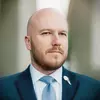
Jared Armes
Courses Plus Student 6,391 PointsThank you! I've added your comment as an answer. Though, I'm not quite sure how it works, I appreciate your help!
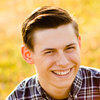
Caleb Kleveter
Treehouse Moderator 37,862 PointsJared Armes , are you wondering how change a comment to an answer?
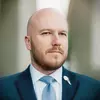
Jared Armes
Courses Plus Student 6,391 PointsCaleb, yes that would be nice to know if it were possible.
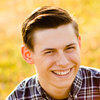
Caleb Kleveter
Treehouse Moderator 37,862 PointsThere are actually a few people who can do that. 1) the poster can cut and paste the comment to an answer, or 2) MOD's can push a button on comments that change them to answers.
2 Answers
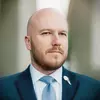
Jared Armes
Courses Plus Student 6,391 PointsA fellow coder, Nat Pham, assisted me. He said that I forgot to use the .each method:
def find_index(name)
index = 0
found = false
todo_items.each do |todo_item|
if todo_item.name == name
found = true
break;
end
index += 1
end
if found
return index
else
return nil
end
end
This solved my problem! Though, I am still unsure as to how it works.
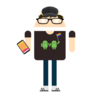
Nhat Pham
14,848 Pointseach method will run a loop i think, and each loop they will return one by one in todo_items array into a block , in this case is | todo_item | <= this called block. And you will learn about block in the next videos . I hope this help
Nhat Pham
14,848 PointsNhat Pham
14,848 PointsI think you're missing .each method to find the index