Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial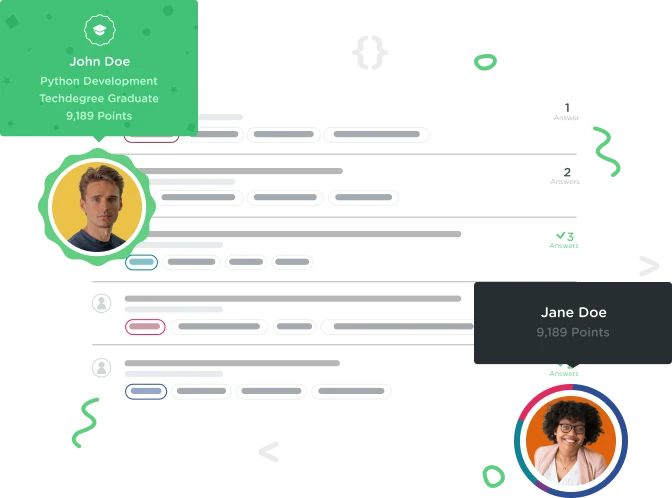

chase singhofen
3,811 Pointsi cant figure out how to make the battery return false if its empty
Create a new public method named isBatteryEmpty that returns true if the battery has 0 bars remaining, and false otherwise. thx
class GoKart {
public static final int MAX_BARS = 8;
private String color;
private int barCount;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty(); {
return isBatteryEmpty == 0;
if(!barCount.isBatteryEmpty());
System.out.println("is not empty");
}
}
3 Answers

Sai Nanduru
1,997 PointsNeed to check, whether barCount variable value is 0 or not. If it is 0, then battery is empty and return true. Otherwise, return false.
public boolean isBatteryEmpty(){ return barCount==0?true:false; }
Note: barCount==0, returns either true or false. Making use of terinary Operator (?) in Java to get the return boolean value

Sean Heaslip
4,490 PointsI feel your pain! I'm learning java basics myself and was stuck on this very same question for a while. I hope the below helps!
The return should appear at the end of your code. This is what the method outputs or 'return'(s).
The 'isBatteryEmpty()' method has a return type ... remember the default return value of boolean (primative) is 'false'. In this instance a boolean returns true if barCount ==0 . The method would return false if the condition is not met.
// default value of primative boolean is'false':
public boolean isBatteryEmpty(){ if(barCount ==0) { return true; } }

apoel fc
344 PointsThe correct answer i think is this :
public boolean isBatteryEmpty(){ if(barCount == 0){ return true; }else{ System.out.println("Battery is not empty"); } } }
if barCount is equals to 0 then its true battery is empty otherwise barCount is not equal to zero so Battery is not empty