Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial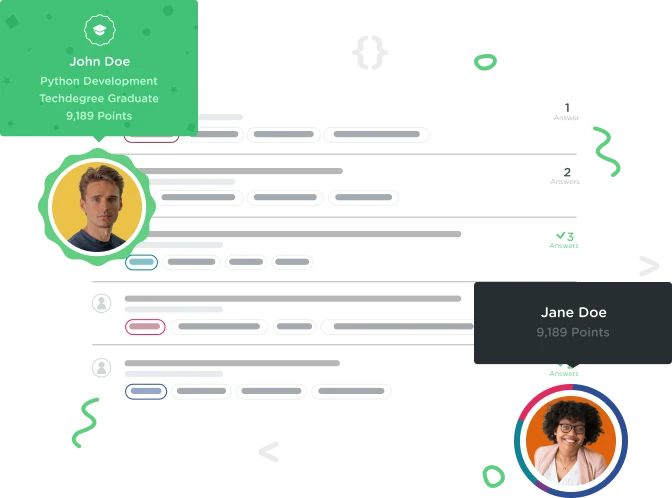
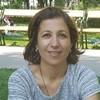
Petya Katsarova
Front End Web Development Techdegree Graduate 21,342 Pointsi cant figure out the way
can someone tell me how to make it dry? i have no idea
const btn1 = document.getElementById("button1");
const btn2 = document.getElementById("button2");
const btn3 = document.getElementById("button3");
const btns = [btn1,btn2,btn3];
function spinElement(event) {
//Applies spinning animation to button element
event.target.className = "spin";
}
const clickBtn1;
const clickBtn2;
const clickBtn3;
.addEventListener('click',spinElement);
<!DOCTYPE html>
<html lang="en">
<head>
<title></title>
<link rel='stylesheet' href='styles.css'>
</head>
<body>
<section >
<button id='button1'>Button 1</button>
<button id='button2'>Button 2</button>
<button id='button3'>Button 3</button>
</section>
<script src='app.js'></script>
</body>
</html>
1 Answer

Luke Pettway
16,593 PointsHello Petya, you are very close to having the answer. The solution lies in using your array of cached elements, btns
. The way that I would go about solving this is looping over that array and then inside of the loop assigning the event listener to each element.
The easiest tool to use would probably be forEach
which can be used to iterate over each of the values in the btns
array giving you access to an individual instance of each item in the current index of the array in the loop.
Below is the solution that I came up with:
const btn1 = document.getElementById("button1");
const btn2 = document.getElementById("button2");
const btn3 = document.getElementById("button3");
const btns = [btn1,btn2,btn3];
function spinElement(event) {
//Applies spinning animation to button element
event.target.className = "spin";
}
// 1. Loop over each btn inside of the btns array (I'm using the name button for each index, but it could be whatever you want)
btns.forEach((button)=>{
// 2. Assign an event listener to the current button
button.addEventListener('click',spinElement);
});
What makes this DRY is that you don't have to do the following:
btn1.addEventListener('click',spinElement);
btn2.addEventListener('click',spinElement);
.......
What's nice is that down the road if you need to add more buttons, you just need to update the array. You could even go a step further with querySelectorAll
and use a class or html attribute to grab all the buttons on a page.