Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial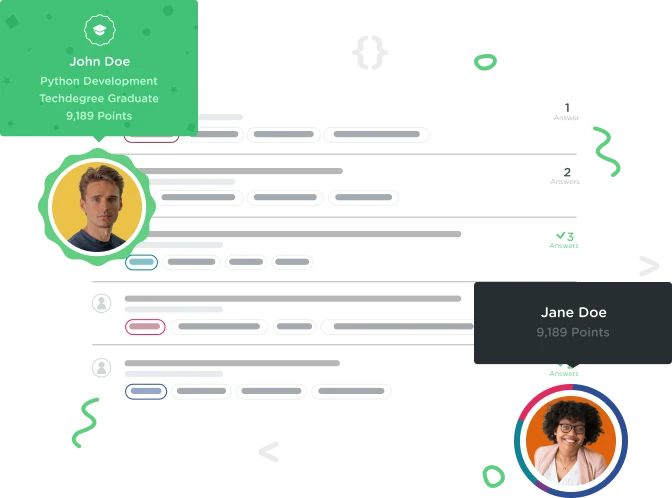

Michael Wilson
1,782 PointsI can't figure out the while statement. Can someone assist me?
There is something wrong with the way I'm writing this code. What is it? Please
``` javascript
var secret = prompt("What is the secret password?"); {
var sesame = secret;
document.write("You know the secret password. Welcome.");
}
while (secret !== sesame) {
document.write("Something is missing.");
}
```index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="app.js"></script>
</body>
</html>
1 Answer

Casey Woelfle
7,603 PointsHi there Michael, There are a few things wrong with your code. Let me explain.
The challenge:
The code in app.js just opens a prompt, asks for a password, and writes to the document. Something is missing. Add a while loop, so that a prompt keeps appearing until the user types "sesame" into the prompt.
The reason your code above cant complete the challenge is because:
- The prompt is not in the loop, and therefore wont continue to pop up until the users enters the correct secret.
- When someone types something in to the prompt, it goes in to our program as a string. So, while we are using our comparison we need to make sure our word is in double or single quotes. For example: "sesame"
- We don't need var sesame = secret; We are using a !== comparison operator in our while loop, so as long as it does not = our secret "sesame" it will not pass. On top of that since sesame is a var and not a string we cant compare it.
So what we do is:
var secret = prompt("What is the secret password?"); //setting the input value to the variable secret
document.write("Something is missing.")
while (secret !== "sesame") {
secret = prompt("What is the secret password?"); //checking to see if it is right, if not... do it again
}
document.write("You know the secret password. Welcome.");// when the password is correct
So, we finished the challenge. I hope this helps you understand where you got stuck, and helps you move forward in your coding goals.
Michael Wilson
1,782 PointsMichael Wilson
1,782 PointsThanks so much Casey! It's beginning to make since.