Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial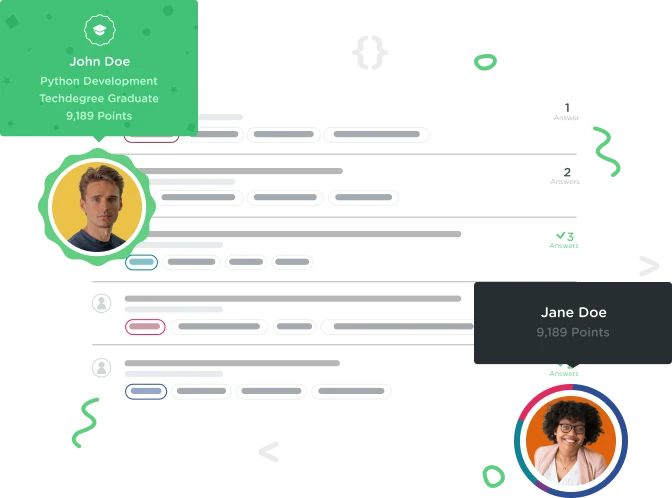

Alastair Herd
2,306 PointsI can't figure out what I am doing wrong.
This is Challenge 4 of Forum from Delivering the MVP.
So close now! Okay so now un-comment the comments in Main.java and Forum.java. PS. You're awesome!
After you uncomment the code in Main.java and Forum.java, fix the code as described in the comments of Main.java. The code in main is attempting to use the code you just fixed.
Unfortunately, I have no idea of what I am doing wrong and so can't move forward. If anyone would be able to shed some light that would be amazing.
Thank you in advance!
public class Forum {
private String topic;
// TODO: add a constructor that accepts a topic and sets the private field topic
public Forum (String topic) {
topic = "";
}
public String getTopic() {
return topic;
}
/* Uncomment this when you are prompted to do so
public void addPost(ForumPost post) {
System.out.printf("A new post in %s topic from %s %s about %s is available",
topic,
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle()
);
}
*/
}
public class User {
// TODO: add private fields for firstName and lastName
private String firstName;
private String lastName;
public User(String firstName, String lastName) {
// TODO: set and add the private fields
this.firstName = firstName;
this.lastName = lastName;
}
// TODO: add getters for firstName and lastName
public String getFirstName() {
return firstName;
}
public String getLastName() {
return lastName;
}
}
public class ForumPost {
private User author;
private String title;
private String description;
// TODO: add a constructor that accepts the author, title and description
public void ForumPost (User author, String title, String description) {
this.author = author;
this.title = title;
this.description = description;
}
public User getAuthor() {
return author;
}
public String getTitle() {
return title;
}
public String getDescription() {
return description;
}
}
public class Main {
public static void main(String[] args) {
System.out.println("Beginning forum example");
if (args.length < 2) {
System.out.println("Usage: java Main <first name> <last name>");
System.err.println("<first name> and <last name> are required");
System.exit(1);
}
// Uncomment this when prompted
Forum forum = new Forum("Java");
// TODO: pass in the first name and last name that are in the args parameter
String firstName = args[0];
String lastName = args[1];
User author = new User(args[0], args[1]);
// TODO: initialize the forum post with the user created above and a title and description of your choice
ForumPost post = new ForumPost(user.getAuthor, user.getTitle, user.getDescription);
forum.addPost(post);
}
}
1 Answer
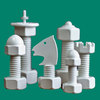
Steven Parker
231,275 PointsThere's a few issues, some of which are with previous tasks. Either the challenge let them slip by or the code got changed later.
First, the instructions for task 1 said to "Initialize the private field topic in the constructor to the value passed in.", but the code here doesn't update the private field. Instead, it sets the parameter to an empty string.
And task 3 asked for a new constructor, but the method "ForumPost" shown above has a type of "void" (constructors don't have a type).
Then the first instruction of task 4 says "now un-comment the comments in Main.java and Forum.java.". In the code above, the comments in "Main.java" have been removed, but those in "Forum.java" still remain.
Finally, the line comments in the newly exposed code in "Main.java" indicate changes that are needed to make the code compatible with the changes made in the previous tasks. This work also still needs to be done.
Alastair Herd
2,306 PointsAlastair Herd
2,306 PointsHi Steven,
Thank you for your reply. I have gone back through and adjusted the things that you mentioned in the first 4 paragraphs (thank you very much for pointing those out)! I think that the challenge did just let them slip, so I'm glad that you helped to correct me.
I'm still struggling on the final line:
I've changed it some what but am still getting this error:
(The ^ arrow is pointed at the new)
Do I need to go back and change something about the User String? Or is it something about the data types that I'm inserting here?
Thank you again for your help.
Steven Parker
231,275 PointsSteven Parker
231,275 PointsThe compiler is trying to convert "J K Rowling" into a "User" object, causing the error. Where it says in the comments "the user created above", it is referring to the variable named "author". So the line would be:
ForumPost post = new ForumPost(author, "Harry Potter", "Childrens Fiction");