Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial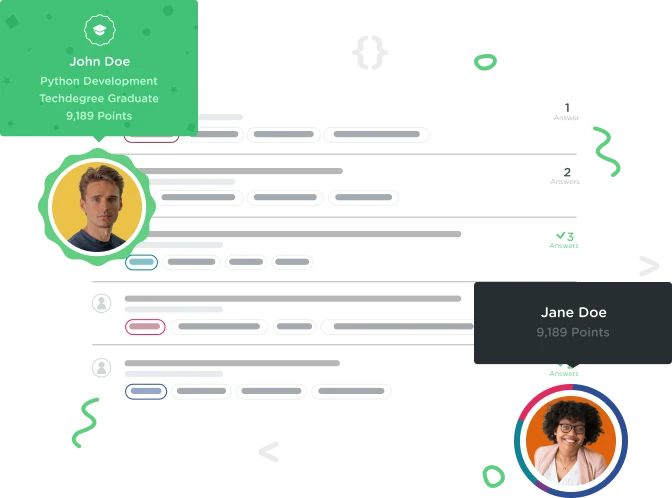

Rafic Rifai
488 PointsI cant figure out what i am doing wrong in the last exercise!! please help
this is my GoKart class
class GoKart { public static final int MAX_BARS = 8; private int barCount; private String color; private int lapsDriven;
public GoKart(String color) { this.color = color; }
public String getColor() { return color; }
public void charge() { barCount = MAX_BARS; }
public boolean isBatteryEmpty() { return barCount == 0; }
public boolean isFullyCharged() { return MAX_BARS == barCount; }
public void drive() { drive(1); }
public void drive(int laps) {
int newAmountOfLaps = lapsDriven + laps;
int newAmountOfBars = barCount - laps;
if ( newAmountOfLaps > newAmountOfBars) {
throw new IllegalArgumentException( "Not enough battery remains");
}
lapsDriven= laps;
}
}
and this is my example class
class Example {
public static void main(String[] args) { GoKart kart = new GoKart("purple"); if (kart.isBatteryEmpty()) { System.out.println("The battery is empty"); } kart.charge(); try { kart.drive(7000); System.out.println("this will never happen"); } catch (IllegalArgumentException iae) { System.out.println("stop right there"); System.out.printf(" the error is %s", iae.getMessage()); }
}
}
the problem is that the exception is not being thrown . i could not find the my mistake.
2 Answers
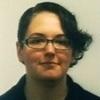
Bryanna Obrien
Courses Plus Student 4,700 PointsThere's not a problem with your code. Just a mismatch between your test and what the challenge wants. Change your kart.drive(7000) back to the original kart.drive(42), and it will run. The challenge just doesn't want you to change that value.
As a side note: your last line 'lapsDriven = laps;'. It works for what the challenge wants, but in reality, you're overwriting the value of lapsDriven every time you want to drive more laps. It would be like the odometer of your car resetting to display just the miles you drove during your last trip instead of the vehicle's total mileage.
Instead of: lapsDriven= laps;
Try: lapsDriven = lapsDriven + laps; or lapsDriven += laps;

Tyson Carlone
2,632 PointsSo i came up with this. It worked and passed the objective. I think it has to do with having two parameters to work with (newLapDriven and newBarCount). If anyone can explain in greater detail why this worked please let me know.
public void drive(int laps) { int newLapsDriven = lapsDriven + laps; int newBarCount = barCount - laps; if (newLapsDriven > MAX_BARS || newBarCount < MAX_BARS){ throw new IllegalArgumentException("Not enough battery"); }