Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial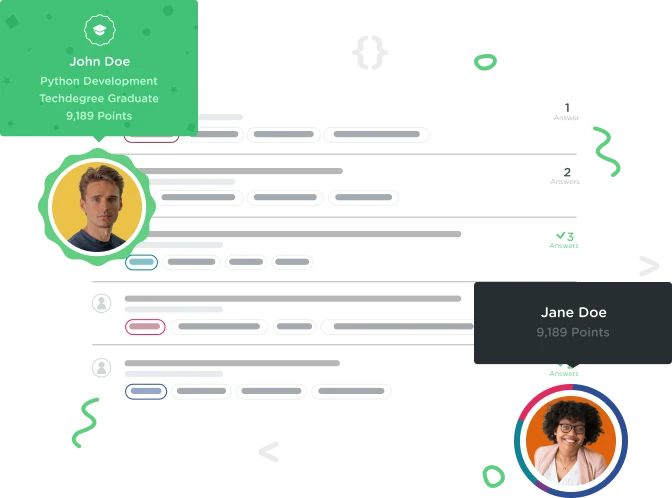

Steve Perry
8,739 PointsI can't figure out what I'm doing wrong. My code looks nearly identical to the videos.
I've pasted in VS and it's telling me numSides doesn't exist in current context.
namespace Treehouse.CodeChallenges
{
class Polygon
{
public readonly int NumSides;
public Polygon(int numSides)
{
NumSides = numSides;
}
}
class Square : Polygon
{
public readonly int SideLength;
public Square(int sideLength) : base(int numSides)
{
SideLength = sideLength;
}
}
Square square = new Square(4);
}
3 Answers

Steve Perry
8,739 Points@Matthew thank you for the thorough response. If the base constructor isn't made for that, then why in the video did he use "x,y" as parameters? When I tried omitting the "int" keyword, it still didn't work for me.
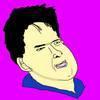
Mathew Tran
Courses Plus Student 10,205 PointsIn the example of the video, the main difference is that the base class required the two parameters for the constructor.
In the case of the square, it is assumed to be 4 sides, and the value wouldn't be anything else or it wouldn't technically be a square. So you would enter the value 4 into the base constructor.
public Square(int sideLength) : base(4)
{
SideLength = sideLength;
}
There are many ways to extend off a class, some classes allow variability and some "lock in" for specific parts -- if that makes sense.
For example.
class Spider
{
public readonly int numberOfLegs;
public readonly int numberOfEyes;
public class Spider(int legs, int numOfEyes)
{
numberOfLegs = legs;
numberOfEyes = numOfEyes;
}
}
class FourLeggedSpider : Spider
{
public readonly Color hairColor;
public class FourLeggedSpider(Color color, int numOfEyes) : base(4, numOfEyes)
{
hairColor = color;
}
}
Note for my Spider class example. When I created a child class called "FourLeggedSpider", the amount of legs was locked in but the amount of eyes could still be chosen.
Hope this helps.
Matt
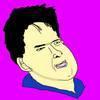
Mathew Tran
Courses Plus Student 10,205 PointsAlso note that in the following code:
public Square(int sideLength) : base(int numSides)
{
SideLength = sideLength;
}
numSides doesn't exist in this context. It isn't being specified in the constructor and the base class object has yet to be created.
You can think of base class as a component with methods / variables, etc.. and child classes build on top of that component.
For example.
A polygon has a number of sides A Square is a Polygon, but is also has sideLength
Square mySquare = new Square(10);
If you were to create a Square in memory somewhere you would see something like this.
=== mySquare instance ===
Square object
--> SideLength: 10
Polygon object
--> Sides : 4
Matt.

Steve Perry
8,739 PointsMathew Tran wow I'm beyond words. This helped a ton man, thanks so much for the thorough response. The Spider example especially illustrated a good point that the video did not. Beyond grateful!
Mathew Tran
Courses Plus Student 10,205 PointsMathew Tran
Courses Plus Student 10,205 PointsYour constructor function for Square is not using the base constructor as it was intended.
Instead of a an integer you've put a variable declaration.
When you inherit from base, it expects values as it would when constructing a Polygon object from scratch.
A good way to remember it, at least for me is how the syntax works when constructing objects.
I hope this makes sense!
Matt