Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial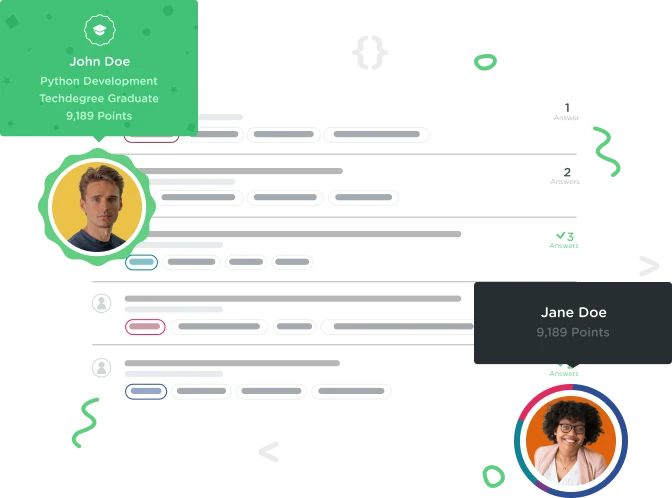

brandonlind2
7,823 PointsI can't figure out why my imported code from the Guns function to the rangedWeapons isnt working, anyone know why?
I have Guns constructor function that I'm importing into the RangedWeapon function, but I don't think its working properly. for example if I delete the Guns function and just put everything in the RangedWeapon function it works correctly. for example if I type rangedWeapons.ar15.damage 80 is returned, however if I create the Guns functions and call it in the RangedWeapon constructor function and type rangedWeapons.ar15.damage, undefined is returned. I'm not sure what I'm writing wrong but if someone could point it out it would be great thanks.
function Ammo(damage,name){
this.damage=damage;
this.name=name;
}
var ammo={
f556: new Ammo(80,'5.56'),
t308: new Ammo(300,'.308'),
n9mm: new Ammo(80,'9mm'),
f45: new Ammo(120,'45acp'),
s76239: new Ammo(150,'7.62x39'),
t22: new Ammo(15,'.22'),
t300win: new Ammo(600,'300win'),
t3006: new Ammo(400, '30-06'),
s76254: new Ammo(400, '7.62x54')
};
function Guns(accuracy,damage,speed,capacity,ammo,range,name){
this.accuracy=accuracy;
this.damage=damage;
this.speed=speed;
this.capacity=capacity;
this.ammo=ammo;
this.range=range;
this.name=name;
}
function RangedWeapon(accuracy,damage,speed,capacity,ammo,range,name){
Guns.call(accuracy,damage,speed,capacity,ammo,range,name);
this.id=0;
this.attachments= [];
this.upgrades= [];
}
RangedWeapon.prototype= Object.create(Guns.prototype);
RangedWeapon.prototype.attachmentAdd= function(attachment){
this.attachments.push(attachment);}
RangedWeapon.prototype.attachmentRemove=function(attachment){
var index= this.attachments.indexOf(attachment);
this.attachments.splice(index,1);
}
RangedWeapon.prototype.upgradeAdd= function(upgrade){
this.upgrades.push(upgrade);}
function Attachment(accuracy,damage,speed,capacity,ammo,range,name){
Guns.call(accuracy,damage,speed,capacity,ammo,range,name);
}
Attachment.prototype= Object.create(Guns.prototype);
var rangedWeapons= {
mdr: new RangedWeapon(90,ammo.t308.damage,80,20,ammo.t308,400,'mdr'),
tavor: new RangedWeapon(70,ammo.f556.damage,90,ammo.f556,200,'tavor'),
ar15: new RangedWeapon(85,ammo.f556.damage,80,30,ammo.f556,300,'AR15'),
ar10: new RangedWeapon(85,ammo.t308.damage,65,20,ammo.t308,400,'AR10'),
akm: new RangedWeapon(60,ammo.s76239.damage,70,30,ammo.s76239,300,'AKM')
};
1 Answer
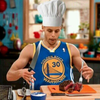
0yzh
17,276 PointsYou need to add 'this' to your .call()
example below:
function RangedWeapon(accuracy,damage,speed,capacity,ammo,range,name){
Guns.call(this,accuracy,damage,speed,capacity,ammo,range,name); // Added 'this' as the first param
this.id=0;
this.attachments= [];
this.upgrades= [];
}
// Some Tests
console.log(rangedWeapons.ar15.damage); // 80
console.log(rangedWeapons.ar15.ammo.name); // 5.56
console.log(rangedWeapons.ar15.range); // 300
brandonlind2
7,823 Pointsbrandonlind2
7,823 Pointsthanks man!
0yzh
17,276 Points0yzh
17,276 Pointsno problemo my friend