Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial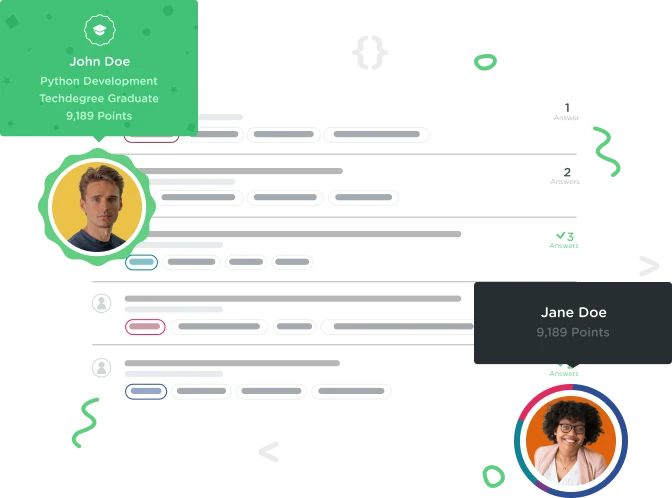
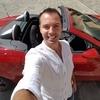
Giulio Vannini
21,922 PointsI can't figure out why this Equals override doesn't work
I believe that this should be working. However it says that the Equals has no virtual/override in its class (which is really weird!) Any idea about what it could be wrong?
using System;
namespace Treehouse.CodeChallenges
{
public class VocabularyWord
{
public string Word { get; private set; }
public VocabularyWord(string word)
{
Word = word;
}
public override string ToString()
{
return Word;
}
public override bool Equals(System.Object w1, System.Object w2)
{
return this.Word == w2.Word;
}
}
}
2 Answers

andren
28,558 PointsThere are multiple issues, first of all the error is technically correct, the method you are trying to override does not exists, you are creating an equals method that takes in two objects, the equals method you inherit from Object only takes one argument, which means they are technically not the same method.
Since the equals method compares the object itself against another object it does not need both of the objects passed in, and in fact you don't even use the w1 argument you created in your own code.
The second issue is that when the object gets passed in to the method it is just that, only an object, it has not been cast to VocabularyWord, which it needs to be in order to access any of the VocabularyWord properties like Word.
The third issue is the fact that you do not check to see if the object is in fact a VocabularyWord object, and not some other object entirely or just null for that matter.
Here is the corrected code with some comments added for clarity:
using System;
namespace Treehouse.CodeChallenges
{
public class VocabularyWord
{
public string Word { get; private set; }
public VocabularyWord(string word)
{
Word = word;
}
public override string ToString()
{
return Word;
}
public override bool Equals(System.Object obj)
{
// returns false if the passed in object is not a VocabularyWord
if (!(obj is VocabularyWord)) {
return false;
}
// Now that we know the object is a VocabularyWord
// We can safely cast it as such
VocabularyWord word2 = obj as VocabularyWord;
// Then we can compare this objects Word with the passed in objects Word
return this.Word == word2.Word;
}
}
}
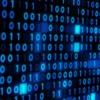
Alexander Davison
65,469 PointsInstead of saying
return this.Word == w2.Word;
Try this instead:
return Word == w2.Word;
This isn't JavaScript! lol
Hope this helps! ~Alex
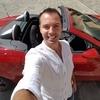
Giulio Vannini
21,922 PointsI still get:
VocabularyWord.cs(18,30): error CS0506: Treehouse.CodeChallenges.VocabularyWord.Equals(object, object)': cannot override inherited member
object.Equals(object, object)' because it is not marked virtual, abstract or override
/usr/lib/mono/4.5/mscorlib.dll (Location of the symbol related to previous error)
Compilation failed: 1 error(s), 0 warnings