Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial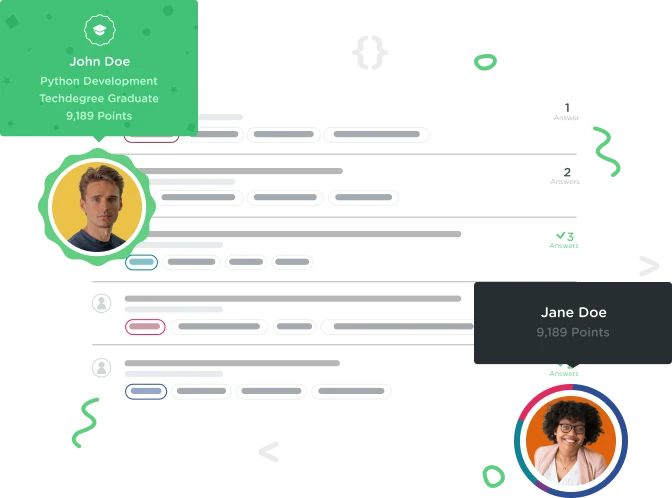

Ryan Allee
278 PointsI can't figure this out can someone please help?
I've tried from what I worked on in the treehouse workspace and used them as a reference, can someone critique my code?
def suggest(product_idea):
return product_idea + "inator"
try:
product_idea = input("Please give name. ")
if product_idea <= 3:
raise ValueError("You'll need more characters than that, sorry.")
except ValueError as err:
print("That is not a real product name")
print("**{}**".format(err))
else:
print("Yes! That's a perfect name!\nThe name is officially: {}".format(ideas))
2 Answers

KRIS NIKOLAISEN
54,971 PointsYou are over complicating it. The first thing to know is a return statement causes the function to end. So you will want to place your code before:
return product_idea + "inator"
Next code will have to be indented to be included in the function.
When I said over complicating there is no need for a try...except...else statements (or input functions). What you need to do is check the length of product_idea and compare that to 3. If it is under then raise the exception. If not then execute your return statement.
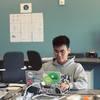
Maxwell Newberry
7,693 PointsOn Line 4, you receive a user's input and subsequently on the next line you check -- I presume -- the length of the input. Something to note with Python, when you use input()
, in Python 2.x or 3.x, the variable type that is returned depends on what the user inputs. For example, if I type "4", then product_idea
's variable type is integer versus if I enter "Maxwell", then the variable type would be string.
You can use raw_input()
to ensure the return variable type is alway **string.
On the next line, you're comparing the inputted text, product_idea
(string), to an integer. This will return an error because comparing a string to an integer cause an unsupported instance. If you wanted to compare the length of the inputted text to check whether or not the text's length is equal to or less than three, you would need to surround the product_idea
variable in the len()
function. This will return an integer of the size of the string. Allowing you to compare the size of the string (integer) to three (integer).
if len(product_idea) <= 3:
I think you may also have mistyped the variable in the final line within the final()
.