Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial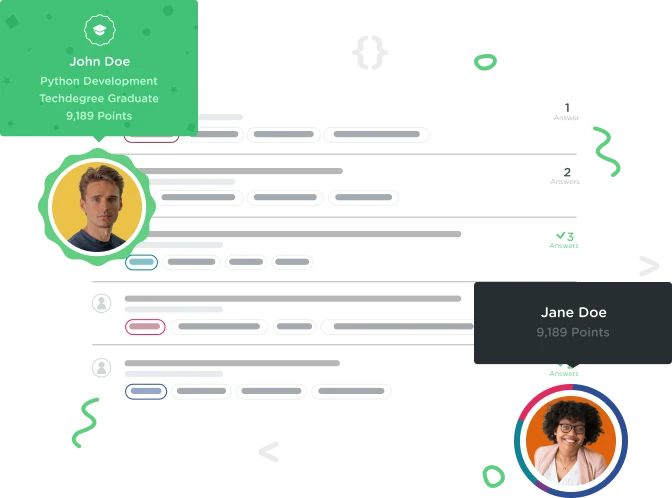
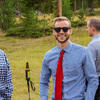
patrick ridd
21,011 PointsI can't find a String.CharacterView method that returns first letter as String in the documentation. Any luck anyone?
I tried casting it to a String but it was a no go. Any ideas on this challenge?
// Enter your code below
extension String {
func modify(function: String -> String) -> String {
return function(self)
}
func firstLetter(word: String) -> String {
let letters = word.characters
let letter = String(letters.first)
return letter
}
}
1 Answer

Greg Kaleka
39,021 PointsHey Patrick,
Couple little issues with your code:
- You need to put that function outside the String extension. We're declaring a function, not a method on String that itself takes a String. Think about how you'd call that, and you'll see why:
"Greg".firstLetter(word: "Greg")
doesn't make sense. Instead, with a plain old function, we just callfirstLetter(word: "Greg")
- You can't assume that the first character is just index 0, because strings are a pain in the
. There are entire WWDC videos explaining why, but here's one example: The character é could be either one or two characters, depending on the encoding (here's a bit of podcast talking about this exact thing). So, instead, you should use the string property
startIndex
.
Here's a solution, not changing much from your code:
// Enter your code below
extension String {
func modify(function: String -> String) -> String {
return function(self)
}
}
func firstLetter(word: String) -> String {
let characters = word.characters
let firstCharacter = characters[word.startIndex]
return String(firstCharacter)
}
In fact, though, we don't need the characters
property at all. We can index directly into the string using startIndex
:
// Enter your code below
extension String {
func modify(function: String -> String) -> String {
return function(self)
}
}
func firstLetter(word: String) -> String {
return String(word[word.startIndex])
}
Cheers
-Greg
patrick ridd
21,011 Pointspatrick ridd
21,011 PointsThanks for your explanation Greg! That helps a ton.
Aditya Ramabadran
3,746 PointsAditya Ramabadran
3,746 PointsGreg Kaleka I think you should explicitly state this
startIndex
stuff in the Question, because due to me not knowing it, this question was a pain in the __ :)Greg Kaleka
39,021 PointsGreg Kaleka
39,021 PointsHey Aditya - I'm just a student like you! Pasan Premaratne might see this and take it into consideration.
For the record, I had to do some research to figure this out myself - Apple documentation, Stack Overflow and Google are your friends
Pasan Premaratne
Treehouse TeacherPasan Premaratne
Treehouse TeacherNoted :) For what it's worth, I do want you to have to struggle through some of these things and look through documentation. We can't make content for every line of code in Swift and iOS; reading documentation and finding answers is an important skill.
Part of that is asking good questions in forums which seems to be happening here :)
Aditya Ramabadran
3,746 PointsAditya Ramabadran
3,746 PointsHaha Greg Kaleka, from your status and the formality of your answer I assumed you were a teacher :)