Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial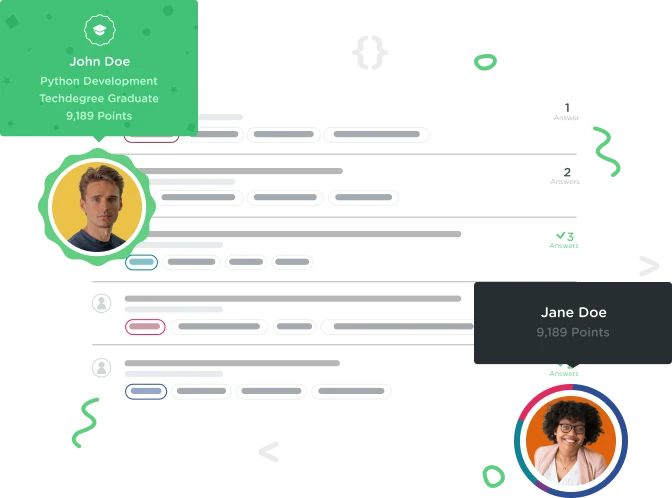

Allan Bakker
607 PointsI can't find an error in my code
There are no errors in the code as far as I can see. I keep getting an error that says value String cannot be null. I tested the code first in visual studio before copying and pasting it here and it worked exactly as I expected it to. Anyone know why it won't work here?
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
while (true)
{
Console.Write("Enter the number of times to print \"Yay!\": ");
string reps = Console.ReadLine();
try
{
int Reps = int.Parse(reps);
if (Reps < 0)
{
Console.WriteLine("You must enter a positive number.");
continue;
}
for (int i = 1; i <= Reps; i++)
{
Console.WriteLine("Yay!");
}
}
catch (FormatException)
{
Console.WriteLine("You must enter a whole number.");
}
break;
}
}
}
}
3 Answers
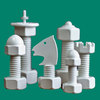
Steven Parker
231,269 PointsYou have an outer loop that prevents the program from ending if bad values are entered..
A program that follows the challenge instructions will only ask for input one time, and either print "Yay!"s or an error message. But either way it should end.
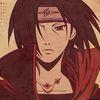
Prateek Jain
Courses Plus Student 1,797 PointsThis was the code I used for the challenge. Maybe it helps to figure out something.
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
int counter = 0;
string times = Console.ReadLine();
try
{
counter = int.Parse(times);
if(counter < 0)
{
Console.WriteLine("You must enter a positive number.");
}
else
{
while(counter > 0)
{
Console.WriteLine("Yay!");
counter = counter - 1;
}
}
}
catch(FormatException)
{
Console.WriteLine("You must enter a whole number.");
}
}
}
}
Since the challenge doesn't ask to repeat the Question if the user enters a wrong input, maybe the while loop that you have used is not required.
Also, you have a continue statement inside the "if condition" but do not have a continue statement inside the catch(FormatException).
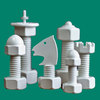
Steven Parker
231,269 PointsA "continue" statement is only allowed inside a loop, so you could not have one. But it's the loop in Allan's code that causes it to fail the challenge.
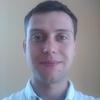
Martin Tesar
3,363 PointsHi, I stuck at final exam for 5 hours. It was hell. Thank you.
And is funny that my solution is working in VS2017 and not her on TTH.
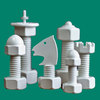
Steven Parker
231,269 PointsThere's a difference between "working" and "performing according to specifications".
Allan Bakker
607 PointsAllan Bakker
607 PointsHey Steven, I now realize that I have indeed put more thought into the program than initially required. The challenge aside is there anything you can think of that would make it not compile since I cant get it to work in the treehouse workspace either. Thanks
Steven Parker
231,269 PointsSteven Parker
231,269 PointsThe code above does compile, it just doesn't pass the challenge due to the outer loop. Your workspace code must be a bit different. You could make a workspace snapshot and post the link to it here and I'll take a look.
Allan Bakker
607 PointsAllan Bakker
607 PointsThanks! I found the error just need to add in the using System;.