Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial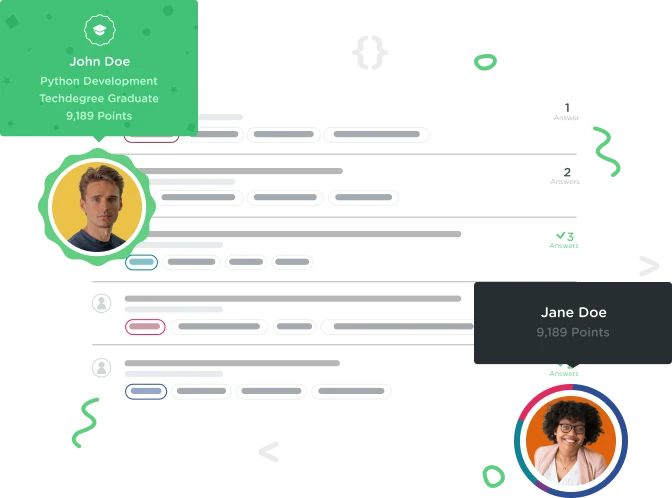
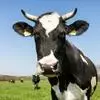
Ava Jones
10,682 PointsI can't get my grid to line up, is anyone able to help?
https://w.trhou.se/dpitc9g5rv <- Snapshot
from cards import Card
import random
class Game:
def __init__(self):
self.size = 4
self.card_options = ["Add", "Boo", "Cat", "Dev", "Egg", "Far", "Gum", "Hut"]
self.columns = ["A", "B", "C", "D"]
self.cards = []
self.locations = []
for column in self.columns:
for num in range(1, self.size + 1):
self.locations.append(f'{column}{num}')
def set_cards(self):
used_locations = []
for word in self.card_options:
for i in range(2):
available_locations = set(self.locations) - set(used_locations)
random_location = random.choice(list(available_locations))
used_locations.append(random_location)
card = Card(word, random_location)
self.cards.append(card)
def create_row(self, num):
row = []
for column in self.columns:
for card in self.cards:
if card.location == f'{column}{num}':
if card.matched:
row.append(str(card))
else:
row.append('')
return row
def create_grid(self):
header = ' | ' + ' | '.join(self.columns) + ' |'
print(header)
for row in range(1, self.size + 1):
print_row = f'{row}| '
get_row = self.create_row(row)
print_row += ' | ' + ' | '.join(get_row) + ' |'
if __name__ == '__main__':
game = Game()
game.set_cards()
game.cards[0].matched = True
game.cards[1].matched = True
game.cards[2].matched = True
game.cards[3].matched = True
game.create_grid()
print(game.create_row(1))
print(game.create_row(2))
print(game.create_row(3))
print(game.create_row(4))
2 Answers
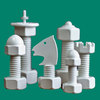
Steven Parker
231,268 PointsYou're close, it just needs a few minor fixups.
When a card is not matched in create_row:
row.append(' ') # same number of spaces an item would take
Then in create_grid:
print_row = f'{row} | ' # adjust spacing
get_row = self.create_row(row)
print_row += ' | '.join(get_row) + ' |' # eliminate extra bar and adjust spacing
print(print_row) # and remember to print the row!
Then finally:
# print(game.create_row(1)) <- none of these are needed because create_grid does it
# print(game.create_row(2))
# print(game.create_row(3))
# print(game.create_row(4))
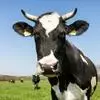
Ava Jones
10,682 PointsI really appreciate your time, working on it now! Thank you.
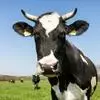
Ava Jones
10,682 PointsIT WORKS, thank you so much!