Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial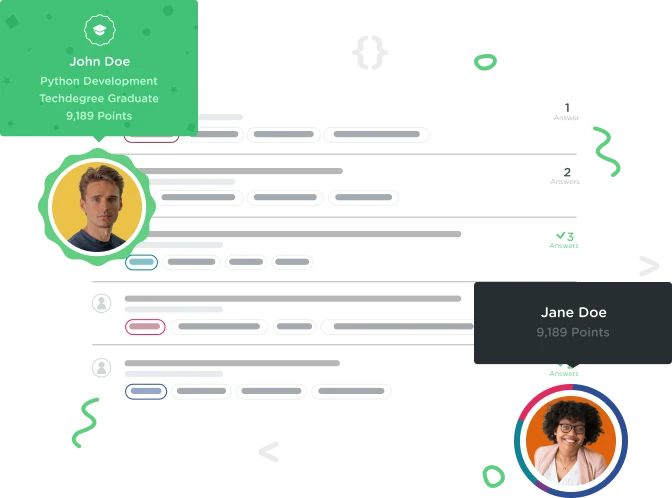

Allan Blain
2,418 PointsI can't get past task 2 I've been trying for days now and haven't seen a right answer in past questions.
This is for task 2 not 1 the bug in the system wouldn't let me use the get help button.
using Treehouse.Models;
namespace Treehouse.Data
{
public class VideoGamesRepository
{
// TODO Add GetVideoGames method
// TODO Add GetVideoGame method
private static VideoGame[] _videoGames = new VideoGame[]
{
new VideoGame()
{
Id = 1,
Title = "Super Mario 64",
Description = "Super Mario 64 is a 1996 platform video game developed and published by Nintendo for the Nintendo 64.",
Characters = new string[]
{
"Mario",
"Princess Peach",
"Bowser",
"Toad",
"Yoshi"
},
Publisher = "Nintendo",
Favorite = true
},
new VideoGame()
{
Id = 2,
Title = "Mario Kart 64",
Description = "Mario Kart 64 is a 1996 go-kart racing game developed and published by Nintendo for the Nintendo 64 video game console.",
Characters = new string[]
{
"Mario",
"Princess Peach",
"Bowser",
"Toad",
"Yoshi"
},
Publisher = "Nintendo",
Favorite = false
}
};
}
}
namespace Treehouse.Models
{
// Don't make any changes to this class!
public class VideoGame
{
public int Id { get; set; }
public string Title { get; set; }
public string Description { get; set; }
public string[] Characters { get; set; }
public string Publisher { get; set; }
public bool Favorite { get; set; }
public string DisplayText
{
get
{
return Title + " (" + Publisher + ")";
}
}
}
}
1 Answer

andren
28,558 PointsSince you haven't posted any code you have attempted to complete the challenge with I'll assume you have no idea how to start solving this task, in that case I will provide the answer and go through it line by line explaining what it does, hopefully that will help you with these types of tasks in the future.
public VideoGame GetVideoGame(int id)
{
foreach(var videoGame in _videoGames)
{
if (videoGame.Id == id) {
return videoGame;
}
}
return null;
}
public VideoGame GetVideoGame(int id)
I assume you are familiar with everything going on in the first line, I am declaring a method called GetVideoGame that returns a VideoGame object and takes one argument of type int.
foreach(var videoGame in _videoGames)
A foreach loop is a loop that will go through all of the elements in an array, each time through the loop the variable you define for the loop (videoGame is the name I use) will be set to one of the elements of the array.
So the first time the loop runs videoGame will be set to the first item in the _videoGames array, the second time it will be set to the second item and so on.
Once the loop has gone through all of the elements it will stop automatically.
if (videoGame.Id == id) {
In this If statement I'm checking to see if the current VideoGame item's Id property equals the id that got passed into the method.
return videoGame;
If the the VideoGame item's Id matches the id that was passed in then I have found the item that was asked for, so I return that right away.
If the current VideoGame item's Id does not match then the loop continues on to the next item in the _videoGames array and runs the If statement again.
return null;
If the loop finishes then that means that none of the VideoGame items in the array had an Id that matched what was passed to the method, so at that point I return null.
If you have any further question about the problem or what I posted then feel free to ask me follow up questions.
Allan Blain
2,418 PointsAllan Blain
2,418 PointsThanks Andren! The system has a bug that won't allow you to submit questions for a seond and I forgot to add my code. Your answer is much appreciated!