Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial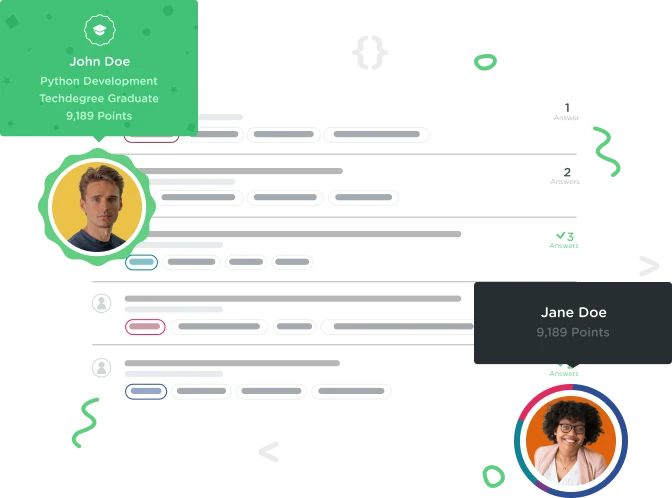

Jake Porritt
10,356 PointsI can't get past this task. I'm struggling to understand Booleans and Javascript in general.
Could someone break it down for me please??
2 Answers
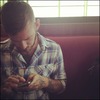
Erik McClintock
45,783 PointsJake,
JavaScript (as well as any other programming language) can be very tricky to understand at first, but don't fret: Everybody feels that way! It's just like trying to learn an actual spoken language, with the caveat that, luckily (and usually), there is more readily available help for programming languages, and it's easier to find people to speak the language with :)
A "boolean" is one of six "primitive data types" in JavaScript (alongside strings, numbers, etc.) that represents the basest logic, in that it is either true or false, and that's it. Programming logic is largely based on true or false values, and that's where the boolean comes in! All you need to remember is: boolean = true or false.
In this particular challenge, they want you to complete the conditional if
statement by passing it a boolean value that will make it evaluate to the first possibility of "This is true". if
conditionals work by this logic: "if(whatever is in these parenthesis is true) { run this first block of code } else { run this block of code! }", so in order to run the first block of code ("This is true", which is what the challenge is asking us to do), we need to a pass a value into the if
conditional to make it evaluate as "true". Since a boolean value is only either true or false, and we need to a) use a boolean value, and b) make it equate to true, we can deduce that we must pass TRUE into the if
conditional!
Thus, your code will look like this:
if( true ) {
alert( 'This is true' );
} else {
alert( 'This is false' );
}
Hopefully that helps to make sense of some things, but let me know if you're still having trouble!
Erik
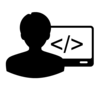
Abe Layee
8,378 PointsThink of Boolean as a switch. A switch is either on or off. In javaScript Boolean is either set to true or false. Also, Boolean is a reserved word which mean you can't not use it as a var like this. Check this site out for list of reserved javaScript words. I hope this was helpful. http://www.quackit.com/javascript/javascript_reserved_words.cfm
var boolean// won't work because is a reserved word.
if (true) {
alert('this is true');
}
else {
alert('this is false');
}