Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial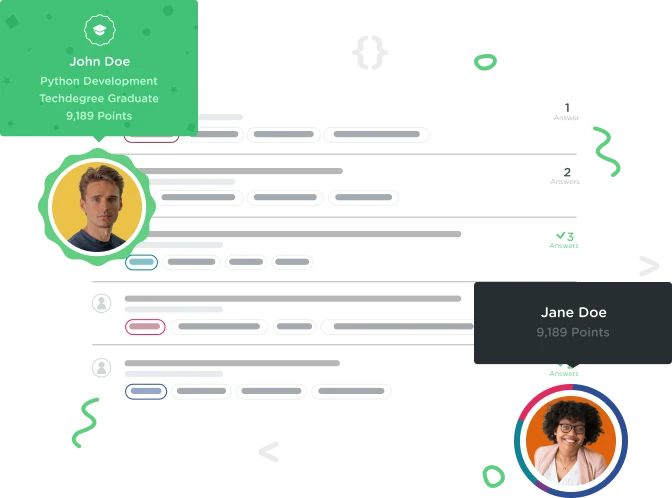

Chokdee Srisuk
19,384 PointsI can't get this challenge passed
This challenge seem trivial to Django view test. We'd like to test the self.article is shown in the article_list view. But I can't figure out what went wrong in the code attached. I think my code is correct. Please help me out.
import datetime
from django.core.urlresolvers import reverse
from django.test import TestCase
from .models import Article, Writer
class ArticleListViewTestCase(TestCase):
'''Tests for the Article list view'''
def setUp(self):
self.writer = Writer.objects.create(
name='Kenneth Love',
email='kenneth@teamtreehouse.com',
bio='Your friendly, local Python teacher'
)
self.article = Article.objects.create(
writer=self.writer,
headline='Article 0',
content='Something about 0',
publish_date=datetime.datetime.today()
)
for x in range(1, 3):
Article.objects.create(
writer=self.writer,
headline='Article {}'.format(x),
content='Something about {}'.format(x),
publish_date=datetime.datetime.today()
)
def articleText(self):
resp = self.client.get(reverse('articles:list'))
self.assertIn(self.articles, resp.context['articles'])
from django.shortcuts import get_object_or_404, render
from .models import Article, Writer
def article_list(request):
articles = Article.objects.all()
return render(request, 'articles/article_list.html', {'articles': articles})
def article_detail(request, pk):
article = get_object_or_404(Article, pk=pk)
return render(request, 'articles/article_detail.html', {'article': article})
def writer_detail(request, pk):
writer = get_object_or_404(Writer, pk=pk)
return render(request, 'articles/writer_detail.html', {'writer': writer})
2 Answers
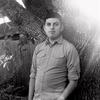
Oziel Perez
61,321 PointsHey i got it to pass, I named the method "test_article_list_view". I don't think that's the issue but just try it anyway. But what's more important is, copy the method, restart the challenge, and paste it back in. Try making sure that the indentation is correct and number of lines in between methods is only one. Remember that Python checks syntax based on indentation and code formatting, so the editor is going to be very picky about this. I know, it sucks, considering that the feedback ("bummer, try again") is terrible.

Anthony Ngene
13,557 PointsHey,
on the last line, self.articles
should be self.article
.
Oziel Perez
61,321 PointsOziel Perez
61,321 PointsI agree, it has to be the correct code. I don't understand why Kenneth's challenges are the only ones that have been so vague about error feedback and so hard to get through.