Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial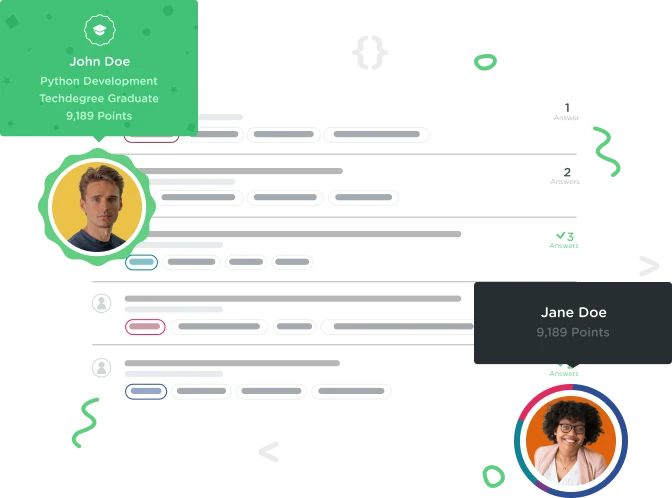

Brennan Altringer
7,660 PointsI can't quite get this one. Anyone have any help they could offer?
I'm not really sure what the question is asking and not sure where my code is wrong. I know I need to be using the list constant but I'm a little lost on this one.
const list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
let thing = e.target.parentNode;
let el = thing.nextSibling;
el.className = 'highlight';
}
});
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<section>
<h1>Making a Webpage Interactive</h1>
<p>Things to Learn</p>
<ul>
<li><p>Element Selection</p><button>Highlight</button></li>
<li><p>Events</p><button>Highlight</button></li>
<li><p>Event Listening</p><button>Highlight</button></li>
<li><p>DOM Traversal</p><button>Highlight</button></li>
</ul>
</section>
<script src="app.js"></script>
</body>
</html>
3 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Brennan,
This is what the html looks like for each list item:
<li><p>Element Selection</p><button>Highlight</button></li>
So what the challenge instructions are saying is that if you click on that button then the paragraph right before it should receive the "highlight" class. The paragraph is a previous sibling to the button.
Putting that together you would have,
e.target.previousSibling.className = "highlight";
e.target is the button element, then we retrieve the prevous sibling which is the paragraph, and then apply the "highlight" class.

Matthew Caloger
12,903 PointsRather than find the parent node, you just want the previousSibling of the clicked button, which would be the paragraph.
const list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
e.target.previousSibling.className = "highlight";
}
});
You were getting the <LI> that contained the button, and then going to the <LI> after the current one and applying highlight on that.
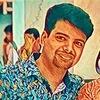
Amrit Pandey
17,595 Pointsconst list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
var previousPara = e.target.previousSibling;
previousPara.className = "highlight";
}
});
This is cleaner and nice to understand, You first select the para preceeding button and then apply the class to it!