Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial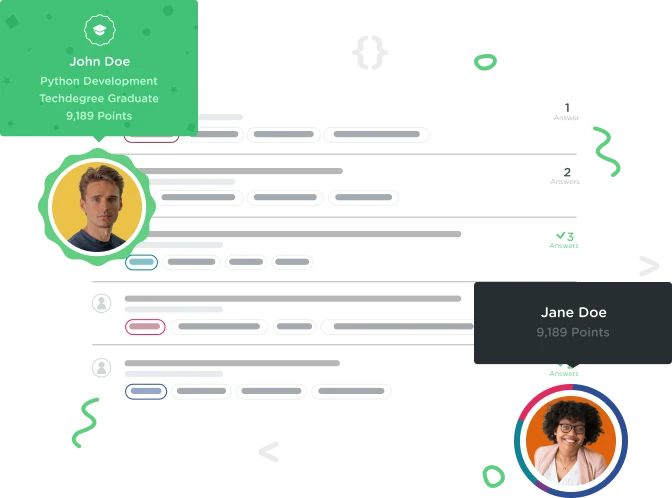

Quinton Dobbs
5,149 PointsI can't rank the correct questions properly!
If you get all 5 of the questions right it works fine. But, if you get 4 or less it will give you the silver medal regardless. I've been trying for about an hour and every time if think I've fixed it it causes a problem with the alert for 0 correct answers.
//question 1//
var correctQs = 0;
var q1 = prompt('What is 1 + 1?');
if (parseInt(q1) === 2) {
correctQs += 1
} else {
alert('The correct answer is 2. You said ' + q1);
}
//question 2//
var q2 = prompt('What is the capital of the U.S.?');
if (q2.toUpperCase() === 'WASHINGTON D.C.') {
correctQs += 1;
} else {
alert('The correct answer is Washington D.C. You said ' + q2);
}
//question 3//
var q3 = prompt('Who is the king of pop?');
if (q3.toUpperCase() === 'MICHAEL JACKSON') {
correctQs += 1;
} else {
alert('The correct answer is Michael Jackson. You said ' + q3);
}
//question 4//
var q4 = prompt('What is 35 x 2?');
if (parseInt(q4) === 70) {
correctQs += 1;
} else {
alert('The correct answer is 70. You said ' + q4);
}
//question 5//
var q5 = prompt('How many hours are there in a day?');
if (parseInt(q5) === 24) {
correctQs += 1;
} else {
alert('The correct answer is 24. You said ' + q5);
}
//final alert//
if (correctQs === 5) {
alert('You got them all correct! You get a gold star!');
} else if (3 <= correctQs <= 4) {
alert('You got ' + correctQs + ' questions correct! You get a silver star!');
} else if (1<= correctQs <=2) {
alert('You got ' + correctQs + ' questions correct! You get a bronze star!');
} else {
alert('You got them all wrong. NO STARS FOR YOU!!!');
}
1 Answer
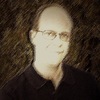
Jason Anders
Treehouse Moderator 145,860 PointsHi...
It's the logical expressions that are a bit weird...
You have the if statement
correct in checking to see if all 5 are correct, but it's the rest that I'm not sure where that syntax came from?
For the first else if
you want to see if there are 3 or 4 correct, so you would use the greater than or equal to
and check it against 3. While 5 is greater than 3, it will never run, because if it is 5, the if
statement would have run and the else if
would be ignored.
The same would go for 1 or 2 correct. You'll want to check and see if it is greater than or equal to
1. Again, 3, 4 and 5 are greater than 1, but this wouldn't be executed if it was.
So, you just need to fix up that logic a bit, as shown below:
if (correctQs === 5) {
alert('You got them all correct! You get a gold star!');
} else if (correctQs >= 3) {
alert('You got ' + correctQs + ' questions correct! You get a silver star!');
} else if (correctQs >= 1) {
alert('You got ' + correctQs + ' questions correct! You get a bronze star!');
} else {
alert('You got them all wrong. NO STARS FOR YOU!!!');
}
Hope this makes sense now. :)
Keep Coding!
Quinton Dobbs
5,149 PointsQuinton Dobbs
5,149 PointsWorks like a charm! Appreciate it. I guess I was just making it more complicated than it needed to be.