Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial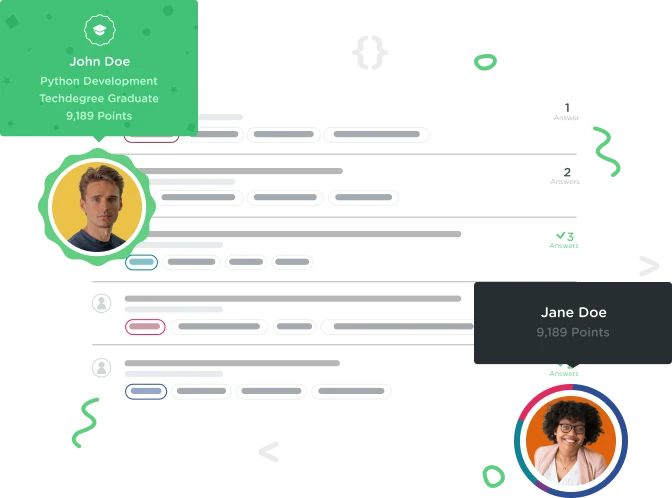

Rasmus Stuckert
1,341 PointsI can't see the problem... Please help
The task:
In the editor you've been provided with two classes - Point to represent a coordinate point and Machine. The machine has a move method that doesn't do anything.
Your task is to subclass Machine and create a new class named Robot. In the Robot class, override the move method and provide the following implementation. If you enter the string "Up" the y coordinate of the Robot's location increases by 1. "Down" decreases it by 1. If you enter "Left", the x coordinate of the location property decreases by 1 while "Right" increases it by 1.
Note: If you use a switch statement you can use the break statement in the default clause to exit the current iteration.
class Point {
var x: Int
var y: Int
init(x: Int, y: Int) {
self.x = x
self.y = y
}
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(_ direction: String) {
print("Do nothing! Im a machine!")
}
}
class Robot: Machine {
override func move(direction: String) {
switch direction {
case "Up": location.y += 1
case "Down": location.y -= 1
case "Left": location.x -= 1
case "Right": location.x += 1
default: break
}
}
}
2 Answers

Dhanish Gajjar
20,185 PointsIt is a very small mistake Rasmus Stuckert, when you overwrote the function, you forgot to keep the underscore ( which omits the external name ). That is all. Keep in mind with these challenges, that in real world situation it is upto you to write functions however you like, but the challenge editor is not forgiving.

Dhanish Gajjar
20,185 PointsIn the class of Machine, do you see the underscore before direction? That is used when you want to omit the external name for a function. The advantage of omitting is that when you call the function move, it will look like
move("Up")
NOT omitting the function, say for example, if the underscore didn't exist, the move function would look like
move(direction: "Up")
When the challenge starts, the move function in the class Machine, has omitted the external name. Therefore, when you create a subclass which inherits the function, should also have the external name omitted. Yours didn't have that.
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(_ direction: String) {
print("Do nothing! Im a machine!")
}
}
Place an underscore before direction
class Robot: Machine {
override func move(_ direction: String) {
switch direction {
case "Up": location.y += 1
case "Down": location.y -= 1
case "Left": location.x -= 1
case "Right": location.x += 1
default: break
}
}
}
Rasmus Stuckert
1,341 PointsRasmus Stuckert
1,341 PointsDhanish Gajjar, what do you mean? Will you please write it in kode?