Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial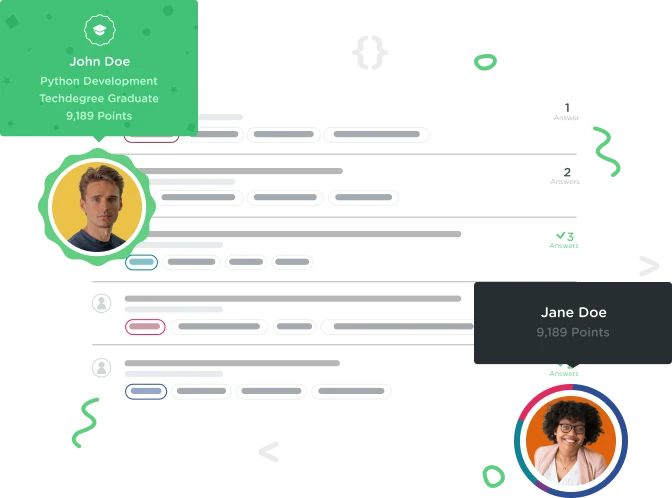
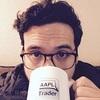
Nicholas Gaerlan
9,501 PointsI can't see what's wrong with my code for the example
99% of the time there's a typo somewhere in my code, I've combed over this so many times and can't find it. I'm just following along with the example in the video but my results are not coming out the same. Can anyone find what I have wrong?
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function () {
if(xhr.readyState === 4) {
var employees = JSON.parse(xhr.responseText);
var statusHTML = '<ul class="bulleted">';
for (var i=0; i<employees.length; i += 1) {
if (employees[i].inoffice === true) {
statusHTML += "<li class="in">";
} else {
statusHTML += "<li class="out">";
}
statusHTML += employees[i].name;
statusHTML += '</li>';
}
statusHTML += '</ul>';
document.getElementById('employeeList').innerHTML = statusHTML;
}
};
xhr.open("GET", "data/employees.json");
xhr.send();
2 Answers
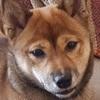
Katie Wood
19,141 PointsYou're really close - the issue is with the addition of the opening <li> tags with the class names - your string is wrapped in double quotes, so when you have a double quote for the class, it's closing the string and causing a javascript error. This results in the list not loading when you preview. The easiest way to fix this is to use single quotes around the whole string, like this:
statusHTML += '<li class="in">';
and
statusHTML += '<li class="out">';
When I copied your code into the workspace, just changing those fixed it - hopefully this gets it working for you!
In the future, it can help to use the JavaScript console in the browser to find errors like this - it won't show anything on the page, but the console will tell you exactly which line is causing the problem, if it's a syntax error.
Opening the browser's JavaScript console is ctrl-J in Chrome, and ctrl-K in Firefox - or open the menu, and it's generally under "Tools" somewhere. I find it really helpful when my JavaScript doesn't run like I expect.
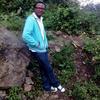
Charles Wanjohi
9,235 PointsThe problem is in use of double quotes in this code:
if (employees[i].inoffice === true) {
statusHTML += "<li class="in">";
} else {
statusHTML += "<li class="out">";
}
}
Always ensure you dont use the double quotes (or even the single quotes) as you have used on class values.The correct code for this is:-
if (employees[i].inoffice === true) {
statusHTML += '<li class="in">'; //note outer quotes are single
} else {
statusHTML += '<li class="out">'; //note the outer quotes are single
}
}
note the other way round would still work i.e outer double quotes and inner single quotes. To use double quotes (or single quotes ) alone, use the escape character before the inner quotes i.e.
if (employees[i].inoffice === true) {
statusHTML += "<li class=\"in\">";
} else {
statusHTML += "<li class=\"out\">";
}
}
Happy coding :)
Nicholas Gaerlan
9,501 PointsNicholas Gaerlan
9,501 PointsThat's it! I was focused on finding a spelling error or an unclosed block or missing semi-colon... that was it! Thanks