Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial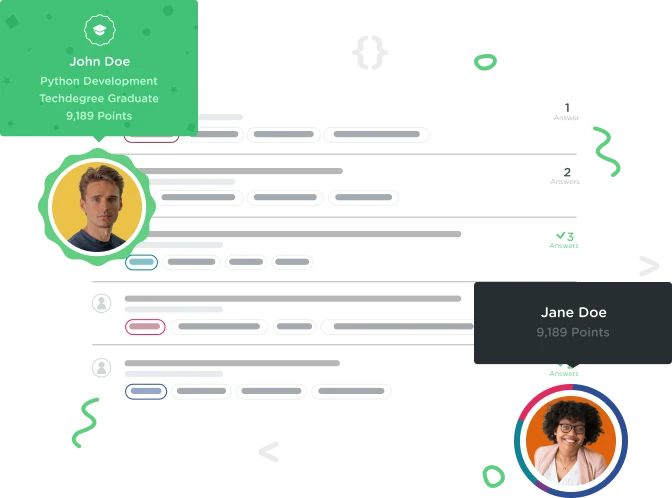
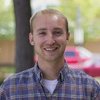
Charles Dunn
5,817 PointsI can't seem to get all the student data to render on the page properly using Dave's method.
I'm having trouble getting all the student data to render on the page properly. It works properly when I use document.write instead of Dave's message variable, but I'm wondering if someone knows what's going on here.
When I console.log(message) it prints all the student data to the console, but only the last student's info actually appears on the page.
var students = [
{
name: 'Brad',
track: 'JavaScript Development',
achievements: 4555,
points: 6899
},
{
name: 'Randy',
track: 'PHP Development',
achievements: 5555,
points: 7899
},
{
name: 'Kelly',
track: 'Python Development',
achievements: 6555,
points: 8899
},
{
name: 'Ethan',
track: 'Ruby Development',
achievements: 7555,
points: 9899
},
{
name: 'Stacy',
track: 'Web Design',
achievements: 8555,
points: 10899
}
];
var message = '';
function print(message) {
var output = document.getElementById('output');
output.innerHTML = message;
}
students.forEach(function(student) {
message = '<h2>Student name: ' + student.name + '</h2>';
message += '<p>Track: ' + student.track + '</p>';
message += '<p>Achievements: ' + student.achievements + '</p>';
message += '<p>Points: ' + student.points + '</p>';
console.log(message);
});
print(message);
7 Answers

Eduard Edwin
14,569 PointsI had the same problem with my code as well. And it all boils down to this line of code:
message = '<h2>Student name: ' + student.name + '</h2>';
It should be written like this:
message += '<h2>Student name: ' + student.name + '</h2>'; // += instead of =
In the former code, every time the loop runs, it initializes a new student to the variable message
instead of adding on to the previous student info.

Iain Simmons
Treehouse Moderator 32,305 PointsOr you could have just changed the first line inside the loop to use +=
:
students.forEach(function(student) {
message += '<h2>Student name: ' + student.name + '</h2>';

ceriannejackson
23,759 PointsHi, you're calling print(message) outside of the foreach loop so it's only being called after the foreach loop has completed. At that point message contains the last student's information.
I think you need to put it in the same place as your console.log(message). That way it will be called at the end of each iteration
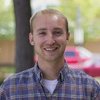
Charles Dunn
5,817 PointsHi @ceriannejackson even with the print function called inside the loop it still only prints out Stacy or whatever the last student in the array is.

ceriannejackson
23,759 PointsDo you need to do += instead of = in this bit:
output.innerHTML = message;
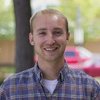
Charles Dunn
5,817 PointsYup that's it! Thanks ceriannejackson !

ceriannejackson
23,759 PointsNo problem :-)