Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial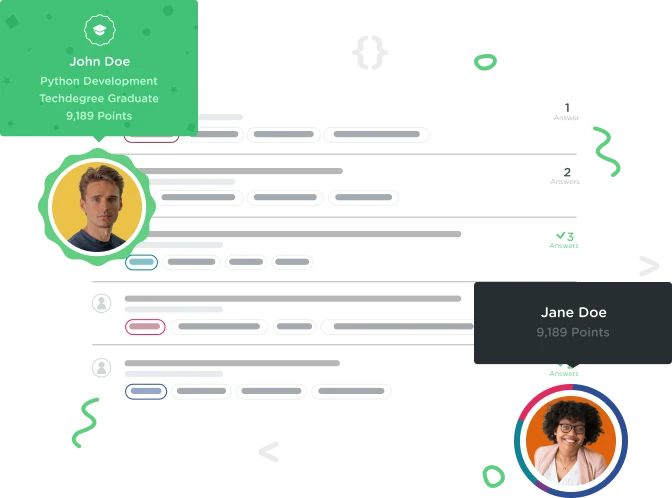
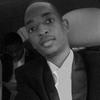
MUZ140998 Clapperton Gwanzura
3,328 Pointsi cant seem to get this passing please help me where am i wrong?
i am creating a new trout object
<?php
class Fish
{
public $common_name;
public $flavor;
public $record_weight;
function __construct($name, $flavor, $record){
$this->common_name = $name;
$this->flavor = $flavor;
$this->record_weight = $record;
}
public function getInfo() {
$output = "The {$this->common_name} is an awesome fish. ";
$output .= "It is very {$this->flavor} when eaten. ";
$output .= "Currently the world record {$this->common_name} weighed {$this->record_weight}.";
return $output;
}
}
class Trout extends Fish{
public $species;
function __construct($name, $flavor, $record, $species) {
parent::__construct($name, $flavor, $record, $species);
$this->species = $species;
}
public function getinfo() {
return $this->species . " " . $this->common_name . " tastes " . $this->flavor . ". The record " . $this->species . " " . $this->common_name . " weighed " . $this->record_weight . "."; ".";
}
$brook_trout = new Trout("Trout", "Delicious", "14 pounds 8 ounces", "Brook");
}
?>
1 Answer

Roberto Rodríguez
48 PointsHi!
You got two errors in your code, let us look at the first error:
Line 26
<?php
parent::__construct($name, $flavor, $record, $species);
?>
The parent constructor method expects three arguments ($name, $flavor and $record), the fourth argument you try to pass ($species) will be ignored and is not required.
Let us look at the second error:
Line 30 to 34
<?php
public function getinfo() {
return $this->species . " " . $this->common_name . " tastes " . $this->flavor . ". The record " . $this->species . " " . $this->common_name . " weighed " . $this->record_weight . "."; ".";
}
$brook_trout = new Trout("Trout", "Delicious", "14 pounds 8 ounces", "Brook");
}
?>
You repeat the variables $species and $common_name and you can not instantiate a class within the same class as you try to instantiate, you need to create the instance to that class out of that class, and you repeated the endpoint ".";
The code would look like this:
<?php
class Fish {
public $common_name;
public $flavor;
public $record_weight;
function __construct($name, $flavor, $record){
$this->common_name = $name;
$this->flavor = $flavor;
$this->record_weight = $record;
}
public function getInfo() {
$output = "The {$this->common_name} is an awesome fish. ";
$output .= "It is very {$this->flavor} when eaten. ";
$output .= "Currently the world record {$this->common_name} weighed {$this->record_weight}.";
return $output;
}
}
class Trout extends Fish {
public $species;
function __construct($name, $flavor, $record, $species) {
parent::__construct($name, $flavor, $record);
$this->species = $species;
}
public function getinfo() {
return $this->species . " " . $this->common_name . " tastes " . $this->flavor . ". The record weighed " . $this->record_weight . ".";
}
}
$trout = new Fish("Trout", "Delicious", "14 pounds 8 ounces");
echo $trout->getInfo();
// The Trout is an awesome fish. It is very Delicious when eaten. Currently the world record Trout weighed 14 pounds 8 ounces.
$brook_trout = new Trout("Trout", "Delicious", "14 pounds 8 ounces", "Brook");
echo $brook_trout->getinfo();
// Brook Trout tastes Delicious. The record weighed 14 pounds 8 ounces.
?>
I hope my explanation has helped you, continues to improve and learn with enthusiasm!
MUZ140998 Clapperton Gwanzura
3,328 PointsMUZ140998 Clapperton Gwanzura
3,328 Pointsthanks hey,got it.