Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial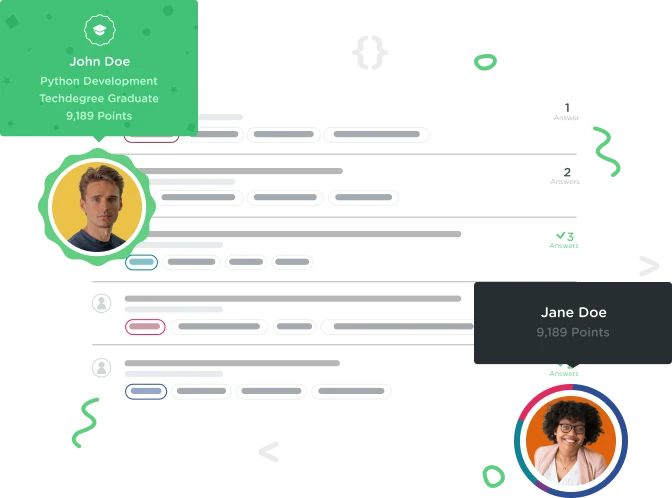

Tom Daugherty
4,821 PointsI can't seem to write this in a way that I return a number?
How do I get this to return a number?
function max (a, b) {
var a = 9;
var b = 5;
if (b > a) {
console.log('the number is '+ 5 +'');
} else {
console.log('the number is '+ 9 + '');
}
}
max ()
1 Answer
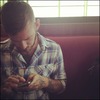
Erik McClintock
45,783 PointsTom,
There are a few things wrong here:
1) You should not be declaring variables and setting values for them in this function. The values that this function will take will be passed into it as arguments when the function is called. So, where you have:
function max (a, b) {
var a = 9;
var b = 5;
// more code below...
You need to remove those var a = 9;
and var b = 5;
declarations:
function max (a, b) {
// more code below...
2) In order to make a function return a value, you need to use the return
keyword. Right now, you are attempting to log to the console, and beyond that, you are not using the value of arguments passed into the function, but rather, you are hard-coding the values of either 5 or 9 into your console.log statements. This defeats the purpose of functions, which is to be reusable chunks of code in varying situations. So, where you have:
function max (a, b) {
if (b > a) {
console.log('the number is '+ 5 +'');
} else {
console.log('the number is '+ 9 + '');
}
}
You need to a) change your console.log
statements to return
statements, and b) you need to remove your hard-coded values, and instead, return the dynamic values that will be supplied by the arguments passed into the function when it is called, which in your case, will be the values held in "a" and "b":
function max (a, b) {
if (b > a) {
return b;
} else {
return a;
}
}
3) Then, when you're on the second task of the challenge, you will be calling the max()
function as the argument for JavaScript's alert()
function, and passing two numbers as arguments (you can use 5 and 9, if you like, or any other two numbers) into your max()
function, making sure they are separated by a comma.
For these concepts that can be very tricky to wrap your head around when you're first starting out, it can help to go back and rewatch the videos a bunch, and even to seek out alternative resources to study with in tandem, like Codecademy, or some great books, like the Head First series from O'Reilly Media. Treehouse is a fantastic resource (the best of all of them, in my experience/opinion!), but if you're not grasping concepts from one resource, it can be very beneficial to branch out. These are basic, core concepts that you need to nail down to be able to program, so do all that you can to make sure you understand them!
Erik