Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial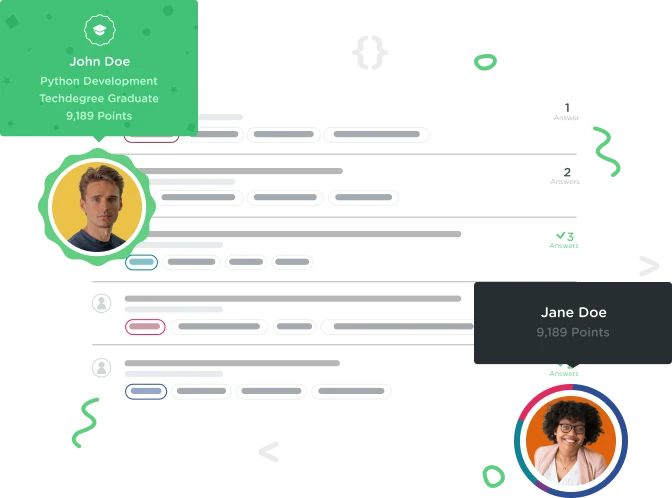

Carly Gloge
429 PointsI can't solve this problem.
I am trying to create a list of numbers for add_list function and then to add them all up with summarize function. I am actually pretty lost - I have no idea if I'm doing this right at all. Could anyone help? Here's my code so far.
number_list = []
def add_list(num):
number_list.append(num)
def summarize():
summarize(add_list)
print("The sum of add_list is {}.".format(summarize(add_list)))
add_list([1,2,3,4,5])
summarize(add_list)
1 Answer

Endrin Tushe
4,591 PointsHi Carly,
This is a good problem to solve. Having a function like this would come in handy, especially when you have large lists that you're trying to sum. So you can actually solve this with just one defined function, by utilizing a for loop. The first thing you have to do is to set the sum = 0. Then you can have a for loop that iterates over each number of the list and adds it to 0. Then you can take that result (or sum) and add it to the next number in the list. Here is what the code would look like:
'''
def add_list(my_list):
sum = 0
for num in my_list:
sum += num
print("The sum of the list is {}".format(sum))
''' So let's say my_list = [1,2,3]
What this code does is the following. The for loop first goes to the number 1, and says hey I need to add this to sum. But sum originally was set to 0 so therefore the sum of 1+0 = 1. This now becomes the new value for sum, so on the next iteration when the for loop goes to the number 2 and says I need to add this to sum, it won't add it to 0 but rather to the new value in sum which is 1. Once it does that the new value of sum becomes 3. Then finally the for loop gets to the last number in the list, 3, and says okay I need to add this to whatever is left in sum (which is 3). So now the sum = 6. You can try this for any list of numbers and it should work.
Carly Gloge
429 PointsCarly Gloge
429 PointsThank you so much, Endrin! I needed to add 'return sum' statement after 'sum += num' for the code to actually execute the addition, but then it passed. I wouldn't have been able to solve the problem without a help. I think setting the sum as 0 at the beginning is the key, but that is difficult for a beginner like me to come up with. I will keep this in mind.