Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial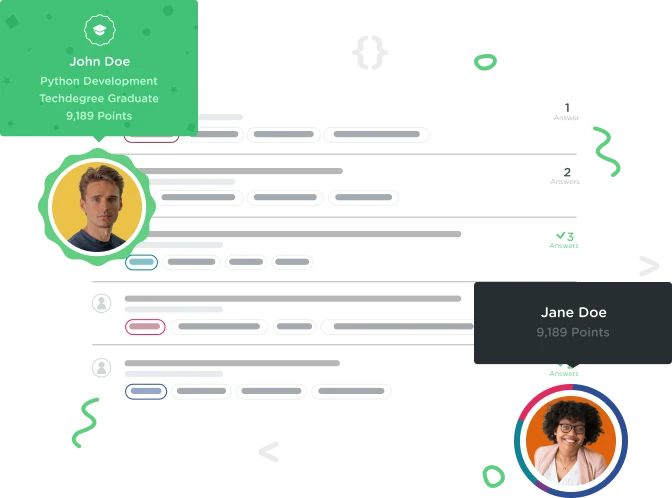

Juan Torres
7,416 PointsI can't tell what's wrong with my code.
when i run it on xcode it comes up with "optional" and then brussels. or something like that.
var europeanCapitals: [String] = []
var asianCapitals: [String] = []
var otherCapitals: [String] = []
let world = [
"BEL": "Brussels",
"LIE": "Vaduz",
"BGR": "Sofia",
"USA": "Washington D.C.",
"MEX": "Mexico City",
"BRA": "Brasilia",
"IND": "New Delhi",
"VNM": "Hanoi"]
for (key, value) in world {
// Enter your code below
switch key
{
case "BEL", "LIE", "BGR": europeanCapitals.append("\(world[key])")
case "IND", "VNM": asianCapitals.append("\(world[key])")
default: otherCapitals.append("\(world[key])")
}
// End code
}
1 Answer
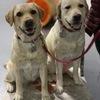
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Juan,
When you perform a dictionary lookup, there's no general guarantee that there will be a value for the specified key. This means that the compiler has to be prepared to handle the case where the result of the lookup is nil
. Because a dictionary lookup can potentially return nil, and because of the way the type system works in Swift, the lookup can only return one type, the type for a lookup has to be Optional(T) (for whatever T is your dictionary value type).
In contrast, when you do for (key, value) in someDictionary
, the compiler knows for certain that every key exists and thus every value exists, so it doesn't need to wrap the values in Optionals.
Your code:
.append("\(world[key])")
is performing the dictionary lookup on world and has to get back an Optional. Since your keys are coming from iterating through your for loop, you know for certain that every key has a corresponding value, so you could (but shouldn't!) force unwrap each lookup to get the underlying value:
.append("\(world[key]!)")
This will pass the challenge (because your output array will comprise Strings instead of Optional Strings) but is very dangerous code, so don't do it!
Instead, remember that In your code, you've specified for (key, value)
when defining your for loop. This means that when you write your case statement, you don't need to lookup a value in the dictionary (which is going to give you value wrapped in an Optional), you already have the value in the constant value
. This means that anytime you write:
.append("\(world[key])")
to access the value and insert it into the array, you could instead just have written:
.append(value)
Hope that's clear
Cheers
Alex