Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial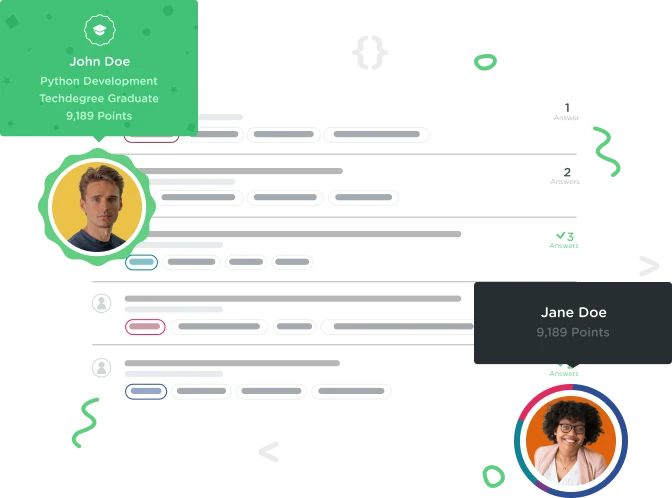

Gal Neeman
1,224 PointsI cant understand what I need to do... =_=
I really dont understand what they want me to do there... Could someone please help me out?
public class GoKart {
private String mColor = "red";
public String getColor() {
return mColor;
}
}
2 Answers

Andrew Winkler
37,739 PointsThe answer above is correct, but I presume that the root of your question likely stems from misunderstanding of what a constructor is/entails. Constructors were concept I had trouble wrapping my head around too, but I'll try to simplify as much as possible.
When an object is created (usually via instantiating a class) any code that you have in your "constructor" will be executed. You don't have to make any special calls – they happen automatically when you create said new object. The constructor method takes the same name as the class it refers to. And it creates several attributes about the object that would otherwise not exist.
The following example is a good demonstration:
public class StudentResults {
private String Full_Name;
private String Exam_Name;
private String Exam_Score;
private String Exam_Grade;
public StudentResults(String name, String grade) {
Full_Name = name;
Exam_Grade = grade;
}
}
The constructor is public StudentResults(String name, String grade) {...}
. Constructor methods take the same name as the class and unlike normal methods, class constructors don't need a return type like int or double (nor any return value for that matter). You can however pass values over your constructors as demonstrated. In the example we've added two String variables to the round brackets of the constructor. Inside the curly braces we've assigned these values to the fields that were previously initialized. That's all I got. Happy coding!
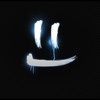
Grigorij Schleifer
10,365 PointsHi Gal,
you need to create a constructor of the GoKart class. The constructor has the same name as the class (GoKart), has no return type and it can take arguments (same as parameters). So our constructor takes the String color as argument. Inside the constructor you will need to assign the privat field mColor to the parameter "color".
Look at this code:
public class GoKart {
private String mColor = "red";
public GoKart(String color) {
// the constructor is public
// has no return type
// and it accepts an String argument "color"
mColor = color;
// assignment of the argument to the mColor field variable
}
public String getColor() {
return mColor;
}
}
Let me know if you need more information :)
Grigorij