Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial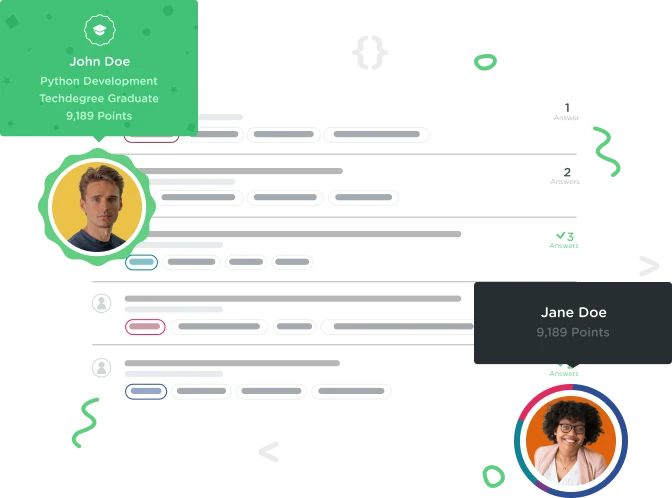

Tommaso Poletti
5,205 PointsI can't understand why use " = " instead " : " inside a object
I can't understand the mechanism that converts
this.name = name || anonnymus;
in
{ name: anonymus }

Tommaso Poletti
5,205 PointsThank you Dennis Brown for responding
2 Answers

Erik Nuber
20,629 Pointsthis.name = name || anonnymus;
{ name: anonymus }
You are only giving part of the code. This.name is apart of a constructor function.
If the value name being passed into that function was not passed in, anonymous would then take it's place. Else the name passed into the function would become this.name
var Cat = function(name, furType) {
this.name = name || "anonymous";
this.furType = furType || "to much fur...help! *koff**koff*";
}
blackCat = new Cat("jasper", "some fur");
whiteCat = new Cat();
Here blackCat would return blackCat.name to be jasper and blackCat.furType would be some fur
And whiteCat.name would be anonymous because nothing was passed in and, whiteCat.furType would be to much fur...help! koffkoff for the same reason
You can copy paste the example I gave into the console and test it yourself to see...
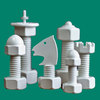
Steven Parker
231,186 PointsI'm not sure what you mean by "converts", these lines don't appear related.
The first line looks like it might come from a constructor function. It would assign an explicit (if defined) or default value to the "name" in a new object being constructed when it is used. But this line by itself doesn't create an object.
The second line is a complete object literal. It exists without being made by a constructor, and has a predefined value for "name".
These lines represent two different ways of assigning a value to a "name" inside an object, but one doesn't "convert" to the other.
Dennis Brown
28,742 PointsDennis Brown
28,742 PointsWhat this is doing is simply assigning the value to the object property directly with dot notation. By doing so when it's read it checks to see if name exists or if 'Portland' exists to use as a default.
We have to use a property in order to use this, because without it we have nothing to reference the parent of. Since we are referencing City with the this keyword, we want to make sure to assign properties to the City object. If we were to use the object literal notation, we would be assigning objects instead of values to the properties.
Another deal-breaker is even though we can use comparison operators in object literals, we cannot use logical operators (or, and, not), at least not effectively.
I hope that clears things up, and if not, I'll be happy to revise what's needed.