Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial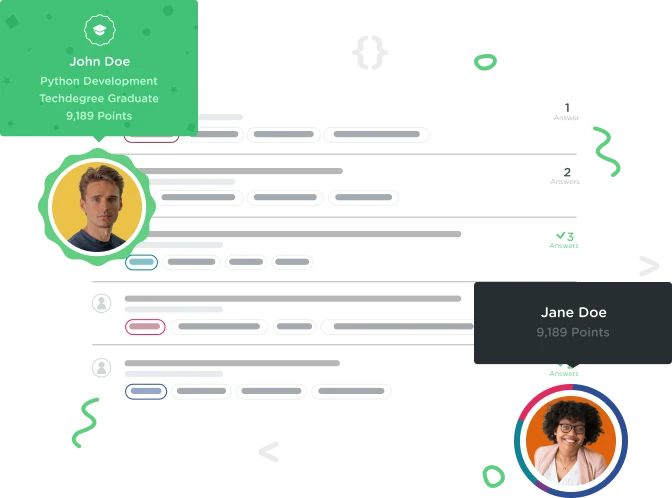
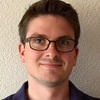
Anthony Lafont
17,075 PointsI can't understand why we don't need to override the initializing method
Hi guys, thanks in advance for helping me.
I can't understand why we don't need to override the initializing method.
Can someone please help me?
class Point {
var x: Int
var y: Int
init(x: Int, y: Int){
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: String) {
print("Do nothing! I'm a machine!")
}
}
// Enter your code below
2 Answers

Florin Veja
6,912 PointsAnthony,
The reason you do not need to override init in this challenge is because it does not ask for the Robot class to have an initial location that differs from what the Machine class, it's parent class, is initialized with. The init method is passed on and accessible from Robot, because it is a superclass of Machine. The location variable gets initialized to x:0, y:0, when you instantiate a Robot class.
In this Challenge, if you just create the Robot class to be a superclass of Machine and not define anything:
class Point {
var x: Int
var y: Int
init(x: Int, y: Int){
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: String) {
print("Do nothing! I'm a machine!")
}
}
// Enter your code below
class Robot: Machine {
}
It is equivalent to saying:
class Point {
var x: Int
var y: Int
init(x: Int, y: Int){
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: String) {
print("Do nothing! I'm a machine!")
}
}
// Enter your code below
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: String) {
print("Do nothing! I'm a machine!")
}
}
Basically, you have the location variable, the init method and the move method. If you want to set self.location to something different then you would want to override init. If you wanted something else to happen when the move method is called, you override the move method.
If the challenge asked for Robot to start from a different location, then it would be necessary to override init. It would also be necessary to override init if the Robot class had defined it's own variables and it needed to initialized them.
Let me know if that makes sense.
Regards,
Florin
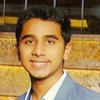
Anish Walawalkar
8,534 PointsSimple answer
Machine is not a subclass of Point, therefore there is nothing to override in the init method
However if you did want to perform some overriding, it would look like this:
class Point {
var x: Int
var y: Int
init(x: Int, y:Int) {
self.x = x
self.y = y
}
}
class Machine: Point {
override init(x:Int, y:Int){
super.init(x: x, y:y)
}
}
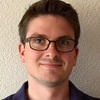
Anthony Lafont
17,075 PointsThanks !
Anthony Lafont
17,075 PointsAnthony Lafont
17,075 PointsThank You Florin, It was very helpy!