Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial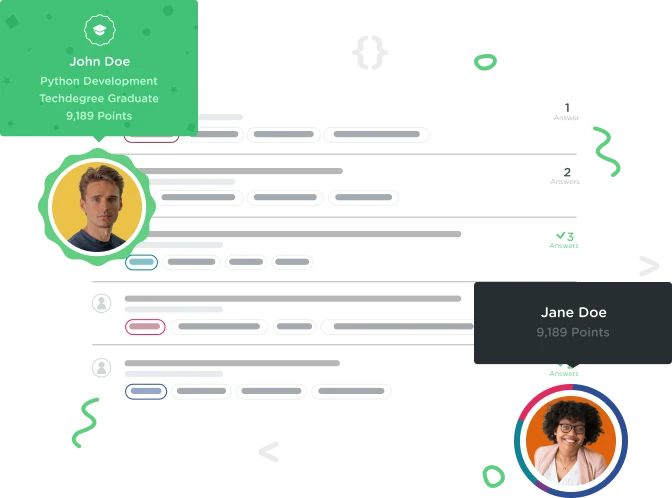
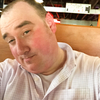
Victor Curtis Jr
5,268 PointsI changed some parameters of the game class and now all the rows and columns are not printing correctly
I was just testing the game class of the card game and I just changed some of the parameters of the rows and columns and now the row and column |
are not printing correctly. Does anyone know how to fix this:
from cards import Card
import random
class Game:
def __init__(self):
self.size = 8
self.card_options = ['Add', 'Boo', 'Cat', 'Dev',
'Egg', 'Far', 'Gum', 'Hut']
self.columns = ['A', 'B', 'C', 'D', 'E', 'F']
self.cards = []
self.locations = []
for column in self.columns:
for num in range(1, self.size + 1):
self.locations.append(f'{column}{num}')
def set_cards(self):
used_locations = []
for word in self.card_options:
for i in range(2):
available_locations = set(self.locations) - set(used_locations)
random_location = random.choice(list(available_locations))
used_locations.append(random_location)
card = Card(word, random_location)
self.cards.append(card)
def create_row(self, num):
row = []
for column in self.columns:
for card in self.cards:
if card.location == f'{column}{num}':
if card.matched:
row.append(str(card))
else:
row.append(' ')
return row
def create_grid(self):
# / A / B / C / D /
header = ' | ' + ' | '.join(self.columns) + ' |'
print(header)
for row in range(1, self.size + 1):
print_row = f'{row}| '
get_row = self.create_row(row)
print_row += ' | '.join(get_row) + ' |'
print(print_row)
def check_match(self, loc1, loc2):
cards = []
for card in self.cards:
if card.location == loc1 or card.location == loc2:
cards.append(card)
if cards[0] == cards[1]:
cards[0].matched = True
cards[1].matched = True
return True
else:
for card in cards:
print(f'{card.location} {card}')
return False
def check_win(self):
for card in self.cards:
if card.matched == False:
return False
return True
def check_location(self, time):
while True:
guess = input(f"What's the location of your {time} card? ")
if guess.upper() in self.locations:
return guess.upper()
else:
print("That's not a valid location. It should look like this: A1")
def start_game(self):
game_running = True
print('Memory Game')
self.set_cards()
while game_running:
self.create_grid()
guess1 = self.check_location('first')
guess2 = self.check_location('second')
if self.check_match(guess1, guess2):
if self.check_win():
print('Congrats!! You have guessed them all!!')
self.create_grid()
game_running = False
else:
input('Those cards are not a match. Press enter to continue')
print('GAME OVER')
What prints to the console is:
Memory Game
| A | B | C | D | E | F |
1| | | | |
2| |
3| | | |
4| | | |
5| |
6| |
7| | |
8| | |
As you can see some |
are missing. How do you fix this?
2 Answers

jb30
44,806 PointsYou could try adding 16 more items to self.card_options
. You currently have 8 different values for the cards and two of each card, so you have 16 total cards. With the change in the number of rows and columns, you have 48 locations, 32 of which have no cards.
Currently, create_row
only adds spaces if there is a card at that location. You could check if there are no cards at that location and append spacing to row
if there are none.
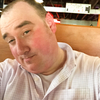
Victor Curtis Jr
5,268 PointsSo I did change the parameters again to print the following:
Memory Game
| A | B | C | D | E |
1| | | | |
2| | | | |
3| | | | |
4| | | | |
5| | | | |
And I did take your advice and changed self.size = 5
and the numbers of cards to 10 and the only thing that is missing is the outer wall for the E
column. Still investigating this.......!?!?

jb30
44,806 PointsIf you run the program multiple times, you are likely to get different gaps in the layout from the empty locations.
You currently have 5 rows and 5 columns, for a total of 25 locations, which is an odd number. With 10 possible values for each card and each card having exactly one match, you have 20 cards total, and 5 locations which do not have cards.
To avoid having empty locations with pairs of cards, multiplying the number of rows by the number of columns should give you twice the length of card_options
. You could try decreasing either self.size
or the number of columns to 4, or increasing self.size
or the number of columns to 6 and adding another 5 values for card_options
.