Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial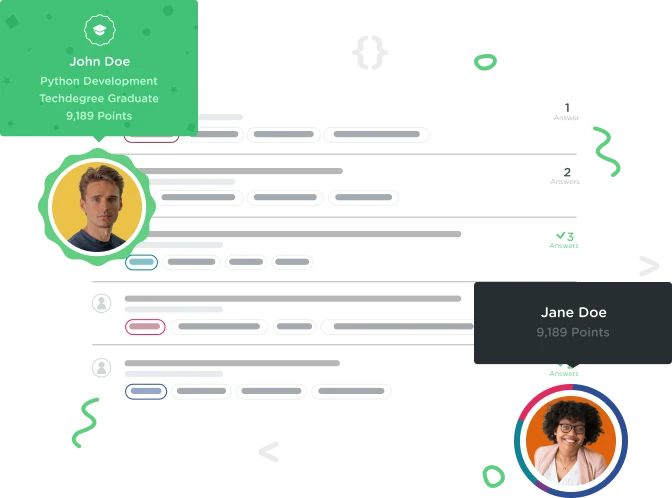

kenweed
12,156 PointsI could not Delete Players, what am I missing?
I've been stuck on this...I got my X's to populate but they won't remove the player names. What am I missing from my code?
Here is my code:
const Header = (props) => { return ( <header> <h1>{props.title}</h1> <span className= "stats">Players: {props.totalPlayers}</span> </header> ); }
const Player = (props) => { return ( <div className = "player"> <span className="player-name"> <button className="remove-player" onClick={ () => props.RemovePlayer(props.id)}>✖</button> {props.name} </span> <Counter/> </div> ); }
//-------------------------------------------------------
class Counter extends React.Component {
state = { score: 0 };
incrementScore= () => { this.setState(prevState => ({ score: prevState.score + 1 })); }
decrementScore = () => { this.setState(prevState => ({ score: prevState.score - 1 })); }
render() { return( <div className="counter"> <button className="counter-action decrement" onClick = {this.decrementScore}> - </button> <span className = "counter-score">{this.state.score}</span> <button className="counter-action increment" onClick={this.incrementScore}> + </button> </div> ); } }
//------------------------------------------------------
class App extends React.Component {
state={
players: [
{
name: "Guil",
id: 1
},
{
name: "Treasure",
id: 2
},
{
name: "Ashley",
id: 3
},
{
name: "James",
id: 4
}
]
};
handleRemovePlayer = (id) => { this.setSate( prevState => { return { players: prevState.players.filter( p => p.id !==id) }; }); }
render(){ return ( <div className="scoreboard"> <Header title = "Scoreboard" totalPlayers = {this.state.players.length} />
{/*PLAYERS LIST */}
{this.state.players.map( player =>
<Player
name = {player.name}
id={player.id}
key = {player.id.toString()}
removePlayer={this.handleRemovePlayer}
/>
)}
</div>
);
}
}
//-----------------------------------------
ReactDOM.render( <App/>, document.getElementById('root') );
3 Answers
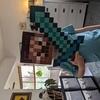
Gabbie Metheny
33,778 PointsHi Ken,
It looks like when you passed the handleRemovePlayer
function to Player
, you typed removePlayer
in camelCase, but when you called it in Player
's event handler, you typed RemovePlayer
in TitleCase. If you just lowercase that R, it should work! Let me know if it doesn't and I can take another look! :)
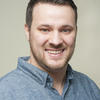
Dwayne Froese
12,930 PointsHi Ken,
Gabbie is correct. You also have a small typo in your handleRemovePlayer function. You seemed to have typed setSate instead of setState.
I copied your code into my editor, made these corrections and ran it and the players could be removed without any problems. Hope this helps!

kenweed
12,156 PointsCORRECTION - Here is my code:
const Header = (props) => {
return (
<header>
<h1>{props.title}</h1>
<span className= "stats">Players: {props.totalPlayers}</span>
</header>
);
}
const Player = (props) => {
return (
<div className = "player">
<span className="player-name">
<button className="remove-player" onClick={ () => props.RemovePlayer(props.id)}>✖</button>
{props.name}
</span>
<Counter/>
</div>
);
}
//-------------------------------------------------------
class Counter extends React.Component {
state = {
score: 0
};
incrementScore= () => {
this.setState(prevState => ({
score: prevState.score + 1
}));
}
decrementScore = () => {
this.setState(prevState => ({
score: prevState.score - 1
}));
}
render() {
return(
<div className="counter">
<button className="counter-action decrement" onClick = {this.decrementScore}> - </button>
<span className = "counter-score">{this.state.score}</span>
<button className="counter-action increment" onClick={this.incrementScore}> + </button>
</div>
);
}
}
//------------------------------------------------------
class App extends React.Component {
state={
players: [
{
name: "Guil",
id: 1
},
{
name: "Treasure",
id: 2
},
{
name: "Ashley",
id: 3
},
{
name: "James",
id: 4
}
]
};
handleRemovePlayer = (id) => {
this.setSate( prevState => {
return {
players: prevState.players.filter( p => p.id !==id)
};
});
}
render(){
return (
<div className="scoreboard">
<Header
title = "Scoreboard"
totalPlayers = {this.state.players.length}
/>
{/*PLAYERS LIST */}
{this.state.players.map( player =>
<Player
name = {player.name}
id={player.id}
key = {player.id.toString()}
removePlayer={this.handleRemovePlayer}
/>
)}
</div>
);
}
}
//-----------------------------------------
ReactDOM.render(
<App/>,
document.getElementById('root')
);