Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial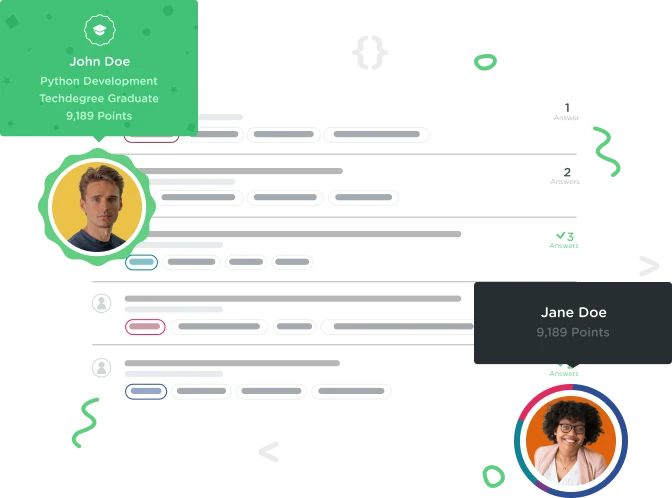

Randell Purington
9,992 PointsI could use some help.
I've provided a base class Person in the editor below. Once an instance of Person is created, you can call fullName() and get a person's full name.
Your job is to create a class named Doctor that overrides the fullName() method. Once you have a class definition, create an instance and assign it to a constant named someDoctor.
For example, given the first name "Sam", and last name "Smith", calling fullName() on an instance of Person would return "Sam Smith", but calling the same method on an instance of Doctor would return "Dr. Smith
I am not sure where I am getting it wrong.
when I click on preview this is what it says:
swift_lint.swift:44:19: error: use of unresolved identifier 'someDoctor' let fullName = someDoctor.getFullName() ^~~~~~~~~~
I have a feeling it is something small I am overlooking but then again I might be way off and not doing what it asks for.
class Person {
let firstName: String
let lastName: String
init(firstName: String, lastName: String) {
self.firstName = firstName
self.lastName = lastName
}
func fullName() -> String {
return "\(firstName) \(lastName)"
}
}
// Enter your code below
class Doctor: Person {
override func fullName() -> String {
return "Dr. \(lastName)"
}
let someDoctor = Doctor(firstName: "Sam", lastName: "Smith")
}
let fullName = someDoctor.getFullName()
1 Answer

andren
28,558 PointsThere are two issues with your current code, but they are indeed both pretty minor issues.
- You are creating the
someDoctor
instance within theDoctor
class, rather than outside the class like you are supposed to. - When you call the method you name it
getFullName
, but the name of the method is actually justfullName
.
If you fix those two issues like this:
class Person {
let firstName: String
let lastName: String
init(firstName: String, lastName: String) {
self.firstName = firstName
self.lastName = lastName
}
func fullName() -> String {
return "\(firstName) \(lastName)"
}
}
// Enter your code below
class Doctor: Person {
override func fullName() -> String {
return "Dr. \(lastName)"
}
}
let someDoctor = Doctor(firstName: "Sam", lastName: "Smith") // Moved outside of Doctor class
let fullName = someDoctor.fullName() // Changed getFullName to fullName
Then your code will pass.
Randell Purington
9,992 PointsRandell Purington
9,992 PointsTHANK YOU!! I appreciate the help. it passed. Thank you!