Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial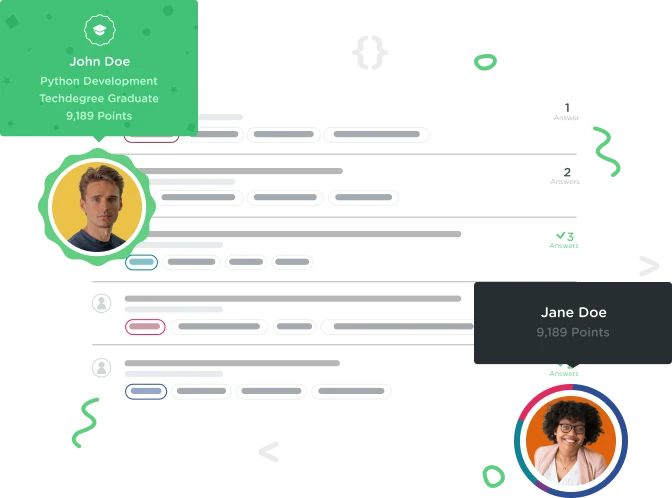

jumper lejava
191 PointsI couldnt get what "->" actually mean. could you please explain?
in the course "methods" in PHP there is expresion "->" e.g.: $p->getinfo() $this->....
i couldn't completely understand what "->" does.
3 Answers
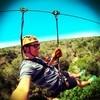
thomascawthorn
22,986 PointsAt it's most basic, it's a way of accessing stuff inside a class. A class can have methods and properties and these can be pulled out by using the ->operator.
Let's say we're talking about you. We could have Your->name, Your->age, Your->phoneNumber.
You would access the information about yourself like this:
<?php
$jumper = new Person;
$jumper->firstName; // 'Jumper'
$jumper->lastName; // 'Lejava'
$jumper->age; // 1040
$jumper->phoneNumber; // 01234123456
and the class of Person would need to look like this.
<?php
class Person {
/**
* @var $firstName string
*/
public $firstName = 'Jumper';
/**
* @var $lastName string
*/
public $lastName = 'Lejava';
/**
* @var $age integer
*/
public $age = 1040;
/**
* @var $phoneNumber integer
*/
public $phoneNumber = 01234123456;
}
Right now, you can think of methods being exactly the same as functions, but you can make them a little cooler. Let's say you wanted to do this:
<?php
$fullName = fullName($jumper->firstName, $jumper->lastName); // 'Jumper Lejava'
and your function would look like this.
<?php
function fullName($firstName, $lastName) {
return $fullName .' '. $lastName;
}
This is something you can do in your object. Add a method like so - again using the ->operator to call a method that belongs to your object.
<?php
$jumper->fullName(); // 'Jumper Lejava'
You class would need to be updated to look like this:
<?php
class Person {
/**
* @var $firstName string
*/
public $firstName = 'Jumper';
/**
* @var $lastName string
*/
public $lastName = 'Lejava';
/**
* @var $age integer
*/
public $age = 1040;
/**
* @var $phoneNumber integer
*/
public $phoneNumber = 01234123456;
/**
* The Persons full name
*
* @return $fullName string
*/
public function fullName()
{
return $this->firstName .' '. $this->lastName;
}
}
Hope this adds to your understanding!
Notice the use of $this. $this refers to THIS very instance of the object. It is a special variable and can't be used for anything else.
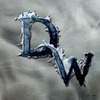
Hugo Paz
15,622 PointsHi Jumper,
The -> is used to access a method or property on an object. Lets say you have the following class:
<?php
class User{
public $name;
public function get_name(){
return $this->name;
}
}
$u = new User;
$u->name = "Peter";
$user_name = $u->get_name();
echo $user_name;
?>
This will echo out "Peter".
Lets explain this step by step:
- You create a class called User with a property (name) and a method (get_name)
- You set the property name to "John".
- The method returns $this->name, this means that if when you create a User object, it will return the object(this) name.
- You create a new user object ($u = new User;).
- You set the object name to 'Peter'.
- You then create a variable which will receive the value of the object name ($user_name = $u->get_name() )
- You then echo the value of the variable.
This is a simple example but i hope you are able to understand it.

Victoria Felice
14,760 PointsGreat explanation. Thank you! Did you correct your example code? I don't see the second step: "You set the property name to "John".
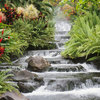
kabir k
Courses Plus Student 18,036 PointsIn addition to the explanation already given, it's called an object operator.
Tony Brackins
28,766 PointsTony Brackins
28,766 Pointswow. This explanation is amazing.