Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial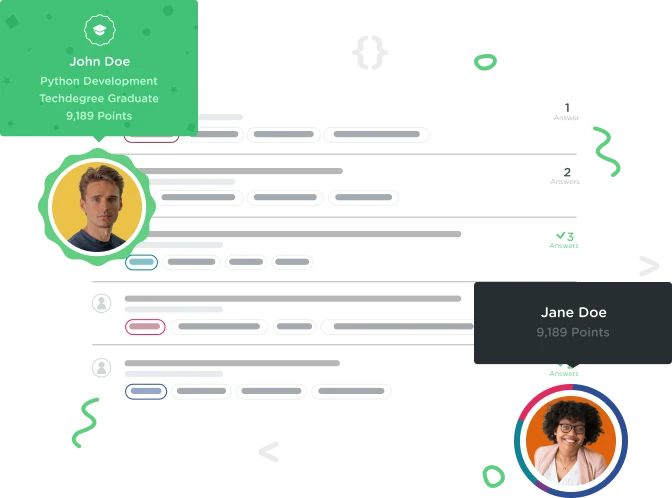

idriss Elouilani
Courses Plus Student 1,232 PointsI create the first function max but is not working
function max (10,6){ var twoNumber = larger of the two numbers; if (the first number >the second number); return true}else {return false }
function max (10,6){
var twoNumbers = larger of the two numbers;
if ( the first number> the second numberlse
}else{ return false;
}
3 Answers
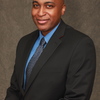
Bryan Knight
34,215 PointsIt could look something like this:
function max(num1, num2){
if(num1 > num2){
return num1;
} else {
return num2;
}
}
You take in two arguments (here I call them num1 and num2). Your conditional checks to see if num1 is greater than num2. If it is then you'll return num1 otherwise (else) return num2.
if you want to get a little fancy with a one liner (ternary) you can do this:
function max(num1, num2) {
return num1 > num2 ? num1 : num2;
}
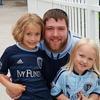
Nick Trabue
Courses Plus Student 12,666 PointsYou want functions to be reusable so your parameters can be called whatever you want.
function max (x,y){
//see if x is larger than y
if (x>y) {
//if it is return x
return x
} else {
//otherwise return y
return y;
}
//Now because it is a function I can reuse it wherever I'd like keeping your code DRY
max(6,4)
//returns 6
max(10,3)
//returns 10
max(10,6)
//returns 10
}

idriss Elouilani
Courses Plus Student 1,232 Pointsthank u Nick; thank u Bryan