Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial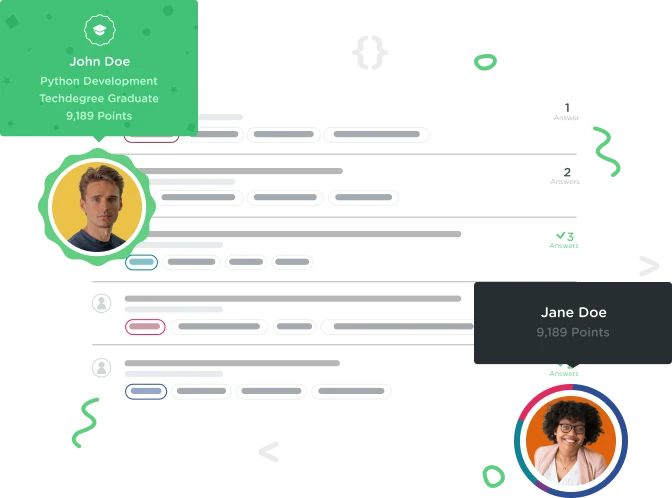

Samuel Cleophas
12,348 PointsI DID IT! Any Suggestions to Improve my Code!?
Hey, so this is the program I wrote for this video. AND IT WORKED. UNBELIEVABLE. I was looking for any suggestions to improve my code - especially by the use of functions or methods I may have forgotten about! Thanks a million!!
Samuel
var quiz = [
['How many aliens have been found thus far?', '0'],
['How many legs does a cocroach have?', '6'],
['What is the last name of the president of America?', 'Trump'],
['How many sides does a square have?', '4'],
['What is the name of the biggest search engine?', 'Google'],
];
var answer = [];
var questionsCorrect = 0;
var HTMLcorrect = "<h2>Correct Answers</h2> <ol>";
var HTMLwrong = "<h2>Wrong Answers</h2> <ol>";
function print(message) {
document.write(message);
}
function wrapInList( item ) {
return "<li>" + item + "</li>";
}
for ( var i = 0; i < quiz.length; i++ ) {
var result = prompt(quiz[i][0]);
answer.push(result);
if ( answer[i] === quiz[i][1] ) {
questionsCorrect += 1;
HTMLcorrect += wrapInList(quiz[i][0]);
} else {
HTMLwrong += wrapInList(quiz[i][0]);
}
}
HTMLcorrect += "</ol>";
HTMLwrong += "</ol>";
print("You got " + questionsCorrect + " questions correct.");
print(HTMLcorrect);
print(HTMLwrong);
Moderator edit: Added JavaScript tag to the markdown so proper syntax highlighting is displayed.
3 Answers
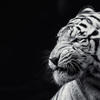
Nick Gentile
4,237 PointsThe one thing I see that might trip it up is case sensitivity (e.g. your answer is 'Trump', what if someone answered 'trump', The toLowerCase method we learned would fix that though....
Also as someone noted above I don't think storing numbers as a string is ever a good idea.
This one tripped me up so I viewed your code and saw you stored your results in an array and used that to compare, which helped me. Very good idea.

Daan Schouten
14,454 PointsLooks good! You could try to turn the 'quiz array' into an object literal, which might look more sophisticated. Object literals consist of key/value pairs. In your case, the quiz question could be the key, and the answer the value. For example:
let quiz = {
"question 1" : 0,
"question 2", 5,
etc..
}
If you haven't gotten to object oriented JS yet, you could just let this code be for a while and try doing it once you get there. It also requires you to make changes in other parts of your code because calling an array is different from calling an object.
Nicely done!

Samuel Cleophas
12,348 PointsHmm. Ok I just learned about that in a later video and I've already done it.. By the way 'let' isn't a key word I've covered in the course.
I was looking for an alternative type of logic / method as opposed to simply following the same logic with different syntax.
Like:
- Is there a better way I can organise my for loop?
- Is there a cleaner way to build the HTML variable? etc.

Daan Schouten
14,454 Pointslet and const are improvements on var, there is actually a fairly easy and straightforward video on treehouse on it: https://teamtreehouse.com/library/let-and-const
As for your other questions, I don't think there is that much that needs changing.