Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial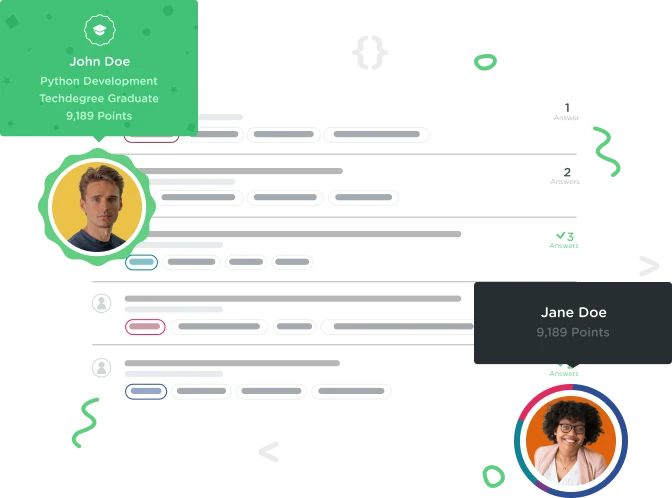

Marina Shumeyko
5,647 PointsI did it. Horaaay!!! Any feedbacks ?
/*
1. Ask questions
2. Store answers
3. Compare user answer with quiz answer
4. If answer is right:
- save it to the variable "correctAnswer";
- print the question;
5. If answer is wrong:
- print the question;
*/
// use to print message
function print(message) {
document.write(message);
}
//ask question
function askQuestion(question) {
answer = prompt(question);
return answer.toLowerCase();
}
//Create array with questions and answers
var quiz = [
['What is the capital of Denmark?', 'copenhagen'],
['What are we learning now?', 'javascript'],
['Do you like to write code', 'yes']
];
var correctAnswer = 0;
var question;
var quizAnswer;
// Print the result
function printResult(arr) {
var correctListHtml = '<h2>You got these question(s) correct:</h2> <ol>';
var wrongListHtml = '<h2>You got these question(s) wrong:</h2> <ol>';
// Compare user answer with quiz answer
for (var i = 0; i < arr.length; i++) {
question = arr[i][0];
quizAnswer = arr[i][1];
if (askQuestion(question) === quizAnswer) {
correctAnswer++;
correctListHtml += '<li>' + question + '</li>';
} else {
wrongListHtml += '<li>' + question + '</li>';
}
}
correctListHtml += '</ol>';
wrongListHtml += '</ol>';
print('You got ' + correctAnswer + ' question(s) right');
print(correctListHtml);
print(wrongListHtml);
}
printResult(quiz);
2 Answers

Tom Lawrence
8,685 Pointslooks good, here is how i did it.
function print(message) {
document.write(message);
}
function runQuiz(questions)
{
var correctAnswers = 0;
for(var i = 0; i < questions.length; i++)
{
var question = questions[i];
var userAnswer = prompt(question[0]);
var answerCorrect = checkAnswer(question, userAnswer);
answerCorrect === true ? correctAnswers++ : correctAnswers = correctAnswers;
}
return correctAnswers;
}
function checkAnswer(question, answer)
{
if(question[1] === answer)
return true;
return false;
}
//start here
var questions =
[
['my name', 'tom'],
['my age', 'unknown'],
['my city', 'newcastle']
];
var correctAnswers = runQuiz(questions);
print(correctAnswers + ' correct');

Marina Shumeyko
5,647 PointsHi Tom. Nice work. I made refactoring in your example, hope it is ok. The main points is:
- no need to create variables, which are do the same work. Try to keep your code simple as much as possible for others. A lot of variables are sometimes can confuse. I was learned about it from JS developers, who's reviewing my code at work.
function print(message) {
document.write(message);
}
function runQuiz(questions) {
var correctAnswers = 0;
for(var i = 0; i < questions.length; i++) {
var userAnswer = prompt(questions[i][0]);
var answerCorrect = checkAnswer(questions[i][1], userAnswer);
answerCorrect === true ? correctAnswers++ : correctAnswers;
}
return correctAnswers;
}
function checkAnswer(question, answer) {
return (question === answer) ? true : false;
}
var questions = [
['my name', 'tom'],
['my age', 'unknown'],
['my city', 'newcastle']
];
print(runQuiz(questions) + ' correct');

Tom Lawrence
8,685 PointsI don't mind, it looks good! always interesting to see how others write the same code.
Mark Wilkowske
Courses Plus Student 18,131 PointsMark Wilkowske
Courses Plus Student 18,131 PointsNice work, Marina! One idea I had and put in my CodePen was this
wrongListHtml += '<li>' + question + ' ' + quizAnswer + ' </li>';
Maybe you want the user to see the answer to a question they got wrong? And how would you color the answer text green?