Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial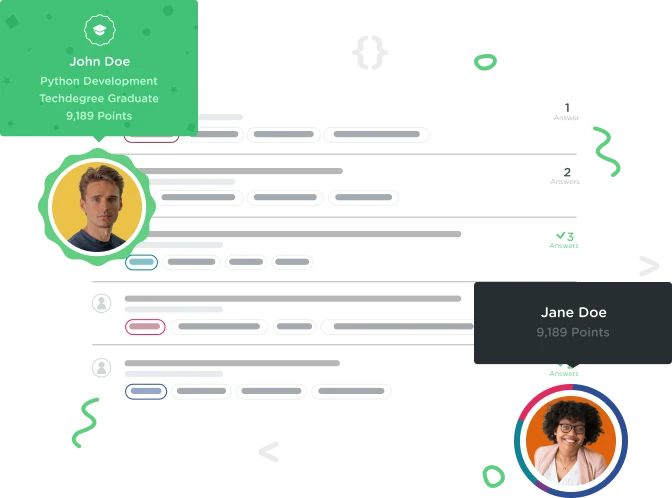

John Gilmer
Courses Plus Student 1,806 PointsI did it right but how does it work?
I think I looked at my code for too long. Now I'm not sure exactly how the console.log(temperatures[i]); portion works! Any explanation would be greatly appreciated! Thanks!
var temperatures = [100,90,99,80,70,65,30,10];
for (var i = 0; i <= temperatures.length; i +=1) {
console.log(temperatures[i]);
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers
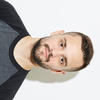
jacobproffer
24,604 PointsHey John,
Each time your for loop runs, the variable i is increased by one. At the same time, the current index of the temperatures array is logged to the console. The index that is logged is determined by the variable i. The loop will continue to run until it reaches the length of the array.
Does that make sense?
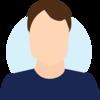
Mike Hatch
14,940 PointsYou can actually slow the loop down, so to speak, to see it in action iteration by iteration. You do this by using the built-in JS object iterator along with the next()
method. Below your current code, you'd type:
const iterator = temperatures[Symbol.iterator]
iterator.next()
Then keep typing iterator.next()
and hit enter after each one to see where the loop is currently at. The output should look like this after each input:
{value: 100, done: false}
{value: 90, done: false}
{value: 99, done: false}
// [etc]
{value: undefined, done: true}
Source: Iterators and generators
Source: Iterators in JavaScript
John Gilmer
Courses Plus Student 1,806 PointsJohn Gilmer
Courses Plus Student 1,806 PointsOH, so it reaches into the array and looks for the index value that I is currently at and since array's start with 0 it can just run down all of the array numbers while logging it. Thank you!