Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial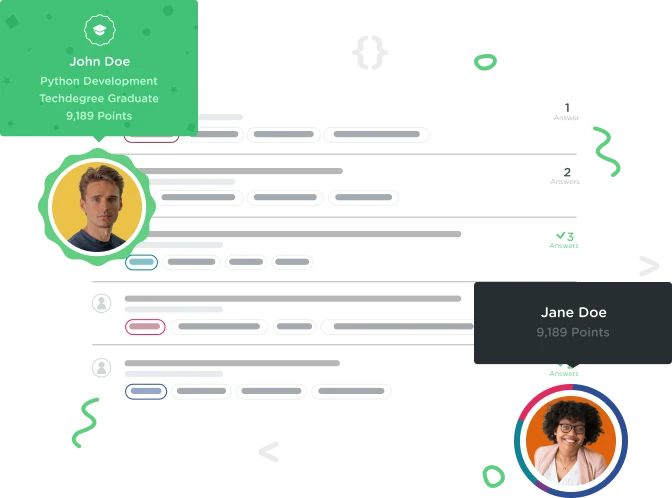
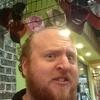
Richard Skinner
5,839 PointsI did it slightly differently. The quiz program works great, but did I forgo "best practices" here?
Let me add my code here. Forgive me, I'm unfamiliar with the markup that actually puts this in a "code box" for clearer reading.
var correct = 0; //logs the number of correct answers
var answer = prompt("How many legs do spiders have? Answer in a number.");
if (parseInt(answer) === 8 ) { //conditional adds 1 to correct answer counter correct += 1; }
answer = prompt("Who discovered the theory of relativity?");
if (answer.toUpperCase() === "ALBERT EINSTEIN" ) { correct += 1; }
answer = prompt("Who was the first president of the United States?");
if (answer.toUpperCase() === "GEORGE WASHINGTON" ) { correct += 1; }
answer = prompt("Who was the famous cofounder of Apple?");
if (answer.toUpperCase() === "STEVE JOBS" ) { correct += 1; }
answer = prompt("Which state's capital is Austin?");
if (answer.toUpperCase() === "TEXAS" ) { correct += 1; }
if (correct === 5) { document.write("<h2>Congratulations! You got all 5 questions right! You win the GOLD CROWN</h2>"); } else if (correct >= 3 && correct < 5 ) { document.write("<h2>Congratulations! You got " + correct + " questions right! You win the SILVER CROWN</h2>");
} else if (correct >= 1 && correct < 2) { document.write("<h2>Congratulations! You got " + correct + " questions right! You win the BRONZE CROWN</h2>");
} else { document.write("<h2>You got no questions right. Sorry, no crown for you.</h2>");
}
So, everything works great, but instead of having a different answer variable for each question, I just reassigned the answer variable. Is that a bad thing to do?
1 Answer
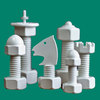
Steven Parker
231,007 PointsThat seems acceptable for the circumstances.
Since each answer is only used on the following line and then never again, it makes sense to re-use the variable. It would also be possible to refactor the code so it doesn't need an answer variable at all.
And instructions for code formatting can be found in the Markdown Cheatsheet pop-up below the "Add an Answer" area.
Richard Skinner
5,839 PointsRichard Skinner
5,839 PointsThanks a lot for your help here. When you say refactor the code where variables are not needed, what do you mean?
The only thing I could immediately think of is putting the prompt function into the conditional test.
Steven Parker
231,007 PointsSteven Parker
231,007 PointsSure, one way would be to use the prompt directly in the if statement. But I was thinking about creating a function to handle the question asking and answer checking that would return 1 for a correct answer and 0 otherwise. Then a good bit of the program becomes more compact and "DRY":