Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial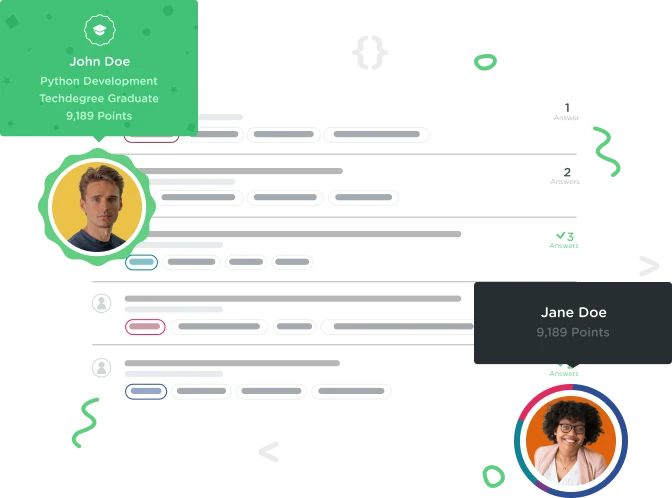

Bei Mi Chen
Full Stack JavaScript Techdegree Student 6,720 PointsI did it two different ways. Which is better? Which is clearer and performs better in browser?
First version:
var students = [
{
name: 'Ben', //Name with string value
track: 'Javascript Full Stack', //eg. iOS, Web Design, Front End Development, Etc.
achievements: 6, // Should hold a number value.
points: 3005, //holds number of points student has earned
},
{
name: 'Danny',
track: 'Python for Data Analysis',
achievements: '3',
points: 118,
},
{
name: 'Hasanda',
track: 'Frontend Development',
achievements: 30,
points: 30000,
},
{
name: 'Tony',
track: 'Backend Development',
achievements: 40,
points: 40000,
},
{
name: 'Luke',
track: 'Java Backend Development',
achievements: 40,
points: 40000,
}
];
function print(message) {
var div = document.getElementById("output");
div.innerHTML = message;
}
function capitalizeFirstLetter(string) {
return string.charAt(0).toUpperCase() + string.slice(1);
}
var studentList = "<ol>";
for(i = 0; i < students.length; i += 1) {
studentList += "<li>"
for ( var key in students[i] ) {
if (key === "name" ) {
studentList += "<strong>" + "Student: "+ students[i].name + "</strong><br>";
continue;
}
studentList += capitalizeFirstLetter(key) + ": " + students[i][key] + "<br>";
}
studentList += "</li>"
}
studentList += "</ol>";
print(studentList);
Second version:
var students = [
{
name: 'Ben', //Name with string value
track: 'Javascript Full Stack', //eg. iOS, Web Design, Front End Development, Etc.
achievements: 6, // Should hold a number value.
points: 3005, //holds number of points student has earned
},
{
name: 'Danny',
track: 'Python for Data Analysis',
achievements: '3',
points: 118,
},
{
name: 'Hasanda',
track: 'Frontend Development',
achievements: 30,
points: 30000,
},
{
name: 'Tony',
track: 'Backend Development',
achievements: 40,
points: 40000,
},
{
name: 'Luke',
track: 'Java Backend Development',
achievements: 40,
points: 40000,
}
];
function print(message) {
var div = document.getElementById("output");
div.innerHTML = message;
}
var studentList = "<ol>";
for(i = 0; i < students.length; i += 1) {
studentList += "<li><strong>Student: " + students[i].name + "</strong><br>";
studentList += "Track: " + students[i].track + "<br>";
studentList += "Achievements: " + students[i].achievements + "<br>";
studentList += "Points " + students[i].points + "<br></li>";
}
studentList += "</ol>";
print(studentList);
2 Answers
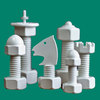
Steven Parker
229,744 PointsBoth methods are clear and perform similarly. But they do different things.
The choice would be a matter of how the database is maintained, and what the viewer needs to see.
If the data items might differ from student to student, the first method would always list all items present.
On the other hand, if the data was intended to remain consistent, the second item would call attention to missing items by listing them as "undefined". Any unexpected items would simply be ignored.

Bei Mi Chen
Full Stack JavaScript Techdegree Student 6,720 PointsThanks everyone! Steven, you bring up very good points. Clearer data structure, right? Evan, you're absolutely right. Code's better when it's readable, not when it's shorter but more complicated. Learning a lot. Any books you guys recommend I should read if I want to become an efficient and effective coder?