Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial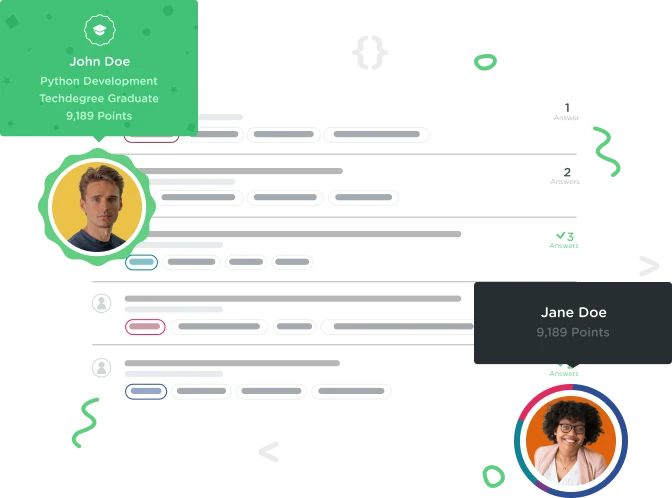

Ali Rasheed
7,180 Pointsi did the code but i used one (for) loop for tracking the answers . is that ok ?
here is how . this code did the same results, is this ok or do i need to make two loops for better programming thinking ?
function print(text){
var theText = document.getElementById("result");
theText.innerHTML = text;
}
var QuestionsAnswers = [
["what is the name of old Iraq?", "mesopotamia"],
["what is the name of the capital", "baghdad"],
["who is kazim alsaher", "singer"]
]
var theResultCorect = "<ol>";
var theResultWrong = "<ol>";
var correct = 0;
var wrong = 0;
alert("take this guiz");
for(var i = 0; i < QuestionsAnswers.length; i++ ){
var answer = prompt(QuestionsAnswers[i][0]);
answer = answer.toLowerCase();
if(answer === QuestionsAnswers[i][1] ){
correct += 1;
theResultCorect += "<li>" + QuestionsAnswers[i][0] + "</li>";
}else {
wrong += 1 ;
theResultWrong += "<li>" + QuestionsAnswers[i][0] + "</li>";
}
}
theResultCorect += "</ol>";
theResultWrong += "</ol>";
var result = "<p>" + "you got " + correct + " question(s) right" +"</p>" ;
result += "<h3>" + "you got these question(s) correct: " + "</h3>" + theResultCorect;
result += "<h3>" + "you got these question(s) wrong: " + "</h3>" + theResultWrong;
print(result);
2 Answers
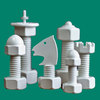
Steven Parker
231,545 PointsYou've combined the list-building with the question-asking. It's not quite as "modular" but it gets the same job done efficiently. For a small program like this one I'd consider it an equally valid solution.
The higher modularity might be more valuable in a larger program.
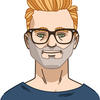
Shane Oliver
19,977 PointsIt's ok in this situation, because the user doesn't get a chance to review or edit questions. But in a situation where they could. You would want to keep the answers in an array and iterate them similarly to what the instructor has done in the video. Here is my take on your answer
var correct = '',
incorrect = '',
result = document.getElementById("result"),
quiz = [
{
question: 'what is the name of old Iraq?',
answer: 'mesopotamia'
}
];
for(var i = 0; i < quiz.length; i++ ){
var question = quiz[i].question
var input = prompt(question);
if( checkAnswer(quiz[i].answer, input) ) {
correct += `<li>${question}</li>`;
continue;
}
incorrect += `<li>${question}</li>`;
}
function checkAnswer(input, answer) {
return input === answer;
}
function print(){
result.innerHTML = `<p>You got ${correct.length} question(s) right.</p>
<h3>You got these question(s) correct:</h3>
<ol>${correct}</ol>
<h3>You got these question(s) incorrect:</h3>
<ol>${incorrect}</ol>`;
}